Kotlin – Write List of Strings as Lines in a File
To write a list of strings as lines in a text file, you can use printWriter()
method. These methods facilitate writing each element of the list to a new line in the file.
In this tutorial, we will show you how to write a list of strings to a file in Kotlin, with each string on a separate line, with an example.
Steps to Write a List of Strings as Lines in a File in Kotlin
To write a list of strings to a text file in Kotlin, follow these steps:
- Import the
File
class from thejava.io
package. - Create a
File
object with the desired file path and name. - Use
printWriter()
method to write each string in the list to a new line in the file.
Kotlin Example Program to Write a List of Strings to a File
In the following example program, we will write a list of strings to a file named 'output.txt'
, with each string on a new line.
Main.kt
import java.io.File
fun main() {
val filePath = "output.txt" // Replace with the desired file path
val lines = listOf("Line 1", "Line 2", "Line 3")
val file = File(filePath)
file.printWriter().use { out ->
lines.forEach { line ->
out.println(line)
}
}
println("List of strings written to file successfully.")
}
Output
List of strings written to file successfully.
The output will confirm that the list of strings has been written to the file, each on a new line.
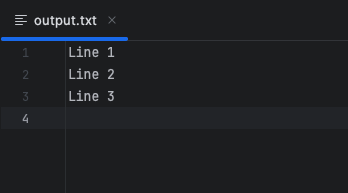
Summary
In this Kotlin tutorial, we learned how to write a list of strings to a text file, with each string as a separate line using File.printWriter()
, with an example.