Android Jetpack Compose – Image
Image composable is used to display an image.
The following is a simple code snippet for Image composable that displays an image resource sample-image.jpg.
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
modifier = Modifier.fillMaxWidth()
)
Example Android Application for Image Composable
In the following example, we display the following image, using an Image composable.
sample-image.jpg
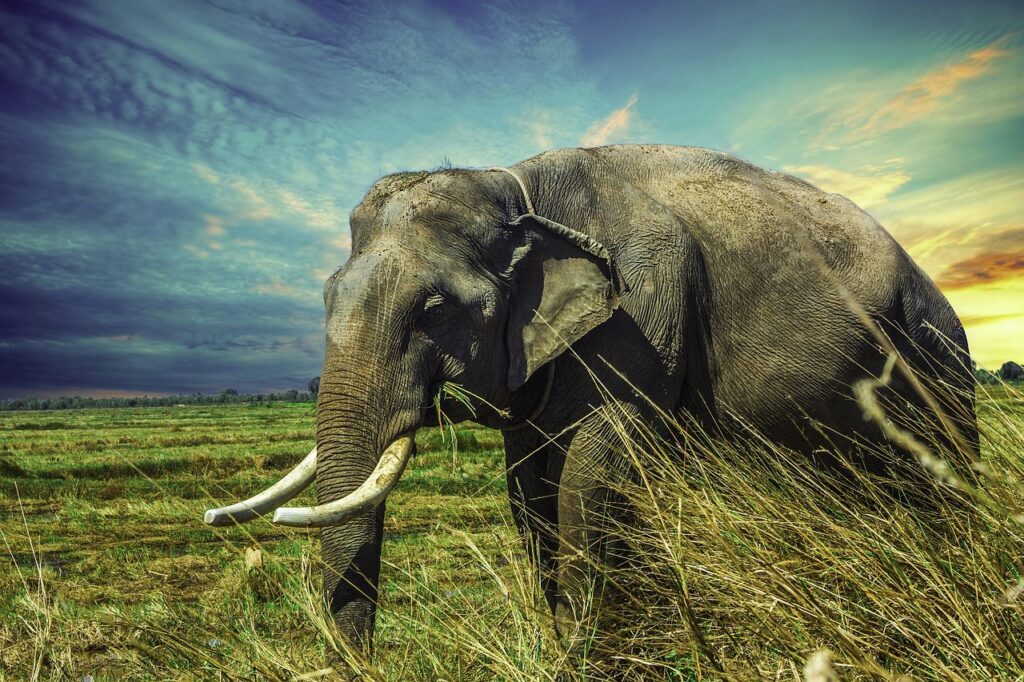
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxWidth().padding(20.dp),
color = MaterialTheme.colorScheme.background
) {
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
)
}
}
}
}
}
Screenshot
Summary
In this tutorial, we have seen how to display in image using Image composable in Android Jetpack Compose, with an example.