Kotlin – Count lines in File
To count the lines in a file in Kotlin, you can use BufferReader to get the file stream, and then use a while loop to read the lines one by one from the stream, and accumulate the count in a variable.
Steps to count lines in File
Follow these steps to find the number of lines in the file.
- Given path to the file in
filePath
variable. - Create a FileReader object using the file path.
- Use BufferReader to get the contents of the file as a stream.
- User readLine() method on the BufferReader object in a while loop, and count the lines in the loop.
Kotlin Program to Count Lines in File
In this example program, we shall take a file 'example.txt'
, and count the lines in it.
The original contents of the 'example.txt'
is given below. There are three lines in this file.
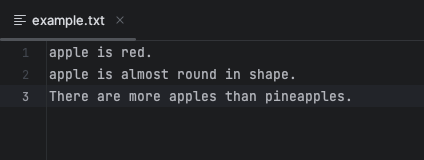
Main.kt
import java.io.BufferedReader
import java.io.FileReader
import java.io.IOException
fun main() {
val filePath = "example.txt" // Replace with the path to your file
var lineCount = 0
try {
BufferedReader(FileReader(filePath)).use { reader ->
while (reader.readLine() != null) {
lineCount++
}
}
} catch (e: IOException) {
println("An exception occurred while reading the file.")
}
println("Number of lines in file : $lineCount")
}
Output
Number of lines in file : 3
There are three lines in given file, and the output reflects the same.
Summary
In this Kotlin File Operations tutorial, we have seen how to count the lines in a file using BufferReader, with a detailed step by step process, and example programs.