Kotlin – Replace String in File
To replace a string in a file in Kotlin, you can read the file, perform the replacement, and then write the modified content back to the file.
Steps to Replace String in File
Follow these steps to replace a specific string with a replacement string in a file given by a file path.
- Import
java.io.File
class. - Given file path in
filePath
variable. - Given search string in
searchString
, and replacement string inreplacementString
variables. We need to replace search string with replacement string in the given file. - Create a
File
object using the file pathfilePath
. - Check if the file exists using the
exists()
method. If the file exists, then read the original content of the file usingFile.readText()
. - Replace the
search
string with thereplacement
string using theString.replace()
function on the original content. - Writes the modified content back to the file using
File.writeText()
.
Example Program to Replace String in File
In this example program, we shall replace the string "apple"
with "cherry"
in the file 'example.txt'
.
The original contents of the 'example.txt'
is given below.
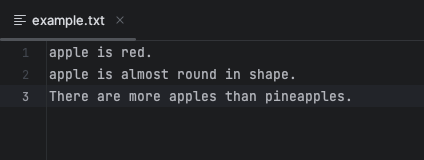
Main.kt
import java.io.File
fun main() {
val filePath = "example.txt" // Replace with the path to your file
val searchString = "apple"
val replacementString = "cherry"
try {
val file = File(filePath)
if (!file.exists()) {
println("File not found: $filePath")
return
}
// Read the file
val originalContent = file.readText()
// Replace the string
val modifiedContent = originalContent.replace(searchString, replacementString)
// Write the modified content back to the file
file.writeText(modifiedContent)
println("String '$searchString' replaced with '$replacementString' in '$filePath'")
} catch (e: Exception) {
println("An error occurred: ${e.message}")
}
}
Output
String 'apple' replaced with 'cherry' in 'example.txt'
example.txt after the execution of program
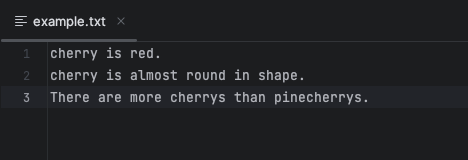
Summary
In this Kotlin File Operations tutorial, we have seen how to replace a string in file using File
class and String.replace()
, with a detailed step by step process, and example program.