Kotlin – Read JSON File
Reading a JSON (JavaScript Object Notation) file in Kotlin can be efficiently achieved using the popular library Gson.
JSON is a common format for data exchange and storage. In this tutorial, we’ll use Gson for simplicity and ease of use.
In this tutorial, we will go through the step by step process of reading a JSON file using Kotlin and Gson.
Setting Up Gson
First, you need to include Gson in your project. If you’re using Gradle, add the following dependency to your build.gradle
file:
dependencies {
implementation 'com.google.code.gson:gson:2.10.1'
}
If you are using IntelliJ IDEA, go to Project Structure, then Project Settings, Modules, under Dependencies, click on the + button, Library, from Maven, and search for ‘com.google.code.gson’. Select the required version, and click on OK, Apply.
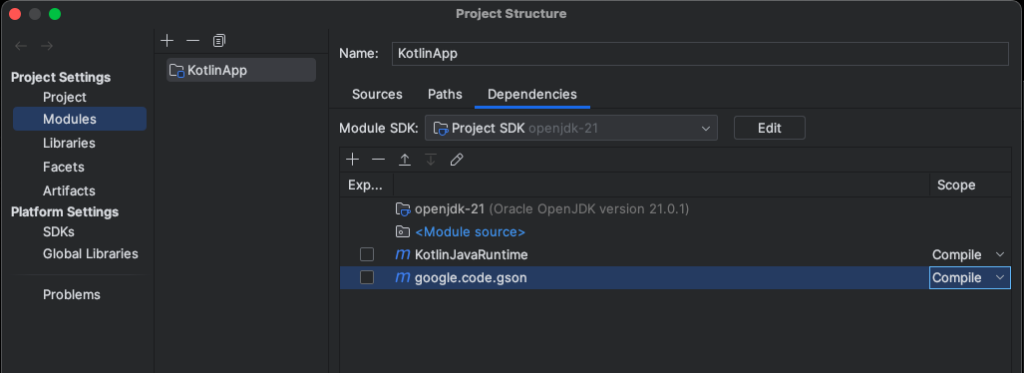
Steps to Read a JSON File in Kotlin Using Gson
To read a JSON file in Kotlin, follow these steps:
- Import the
File
class from thejava.io
package andGson
from the Gson library. - Create a
File
object pointing to your JSON file. - Read the file content into a String.
- Use Gson to convert the JSON string into a Kotlin object or a data class that matches the JSON structure.
Kotlin Example Program to Read a JSON File
Below is an example that demonstrates how to read a JSON file named 'data.json'
and convert it into a Kotlin object:
Assuming you have a JSON file like this.
data.json
{
"name": "Apple",
"age": 30,
"email": "apple@example.com"
}
Create a data class that matches the JSON structure:
data class User(val name: String, val age: Int, val email: String)
Based on the your JSON file structure, you can write this data class file accordingly.
Now, read and parse the JSON file.
Main.kt
import java.io.File
import com.google.gson.Gson
data class User(val name: String, val age: Int, val email: String)
fun main() {
val filePath = "data.json" // Replace with your JSON file path
val file = File(filePath)
val jsonString = file.readText()
val user = Gson().fromJson(jsonString, User::class.java)
println(user)
}
Output
User(name=Apple, age=30, email=apple@example.com)
The output will display the Kotlin object created from the JSON file.
Summary
In this Kotlin tutorial, we demonstrated how to read a JSON file and parse it into a Kotlin object using the Gson library. This method is particularly useful for dealing with JSON data in Kotlin applications.