Android LinearLayout
Android LinearLayout arranges its children horizontally in a row, or vertically in a column.
LinearLayout in XML
LinearLayout tag name is used in layout xml files.
A sample code of LinearLayout in XML layout file is
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:gravity="center">
<!-- child views -->
</LinearLayout>
LinearLayout widget supports many attributes. In the above code snippet, we have given some of them: layout_width, layout_height, orientation and gravity. We will go through each one of them with examples.
Example – LinearLayout
Let us create an Android Application with Kotlin support, and use the following code activity_main.xml layout file.
We have a LinearLayout with vertical orientation and five children. The children are text views.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25sp"
android:textSize="25sp"
android:background="#F5F5F5"
android:text="Item 1" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25sp"
android:textSize="25sp"
android:background="#FFFFFF"
android:text="Item 2" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25sp"
android:textSize="25sp"
android:background="#F5F5F5"
android:text="Item 3" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25sp"
android:textSize="25sp"
android:background="#FFFFFF"
android:text="Item 4" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="25sp"
android:textSize="25sp"
android:background="#F5F5F5"
android:text="Item 5" />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
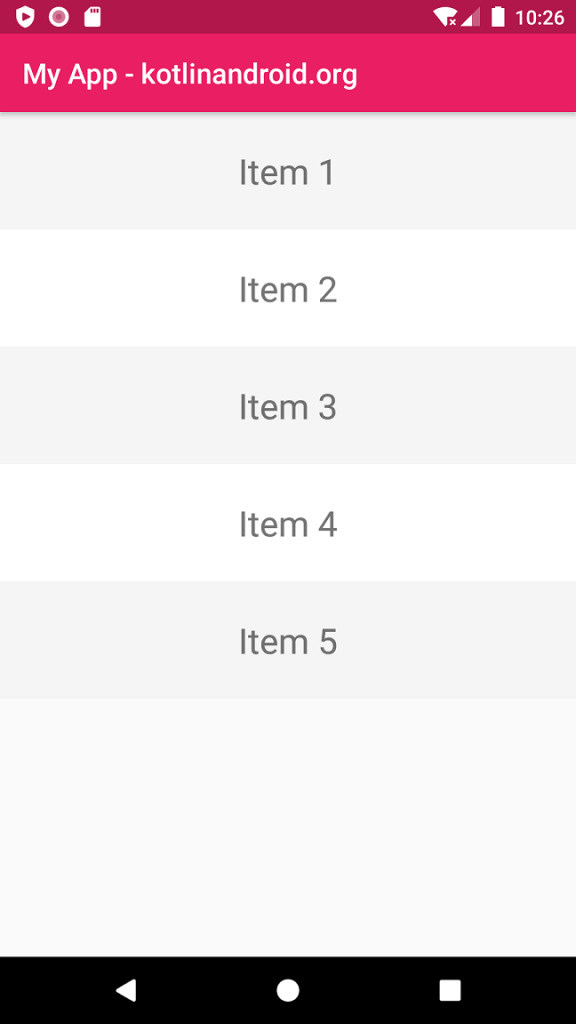
Android Linear Layout Tutorials
To vertically align LinearLayout, read