Kotlin – Check if File is Empty
To check if a file is empty in Kotlin, you can use the length()
method provided by the java.io.File
class. If the length of the file is zero, it means the file is empty.
In this tutorial, we shall see a detailed step by step process to check if file at give file path is empty or not, with examples.
Steps to Check if File is Empty
Follow these steps to check if file at given file path is empty or not.
- Import
java.io.File
class. - Given file path in
filePath
variable. We need to check if this file is empty or not. - Create a
File
object using the file pathfilePath
. - Check if the file exists using the
exists()
method. - If the file exists, use the
length()
method to get the size of the file and compare it to zero to determine if the file is empty. - Print the appropriate message based on whether the file is empty or not.
Example Program to check if File is Empty
In this example program, we shall check if the file 'example.txt'
is empty or not.
If you observe in the following screenshot, the size of the given file is zero bytes.
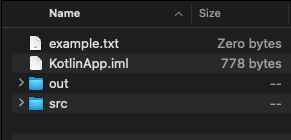
Main.kt
import java.io.File
fun main() {
val filePath = "example.txt" // Replace with the path to your file
val file = File(filePath)
if (file.exists()) {
if (file.length() == 0L) {
println("The file '$filePath' is empty.")
} else {
println("The file '$filePath' is not empty.")
}
} else {
println("The file '$filePath' does not exist.")
}
}
Output
The file 'example.txt' is empty.
Now, let us take a file 'example_1.txt'
which is of size 11 bytes, i.e., not empty.
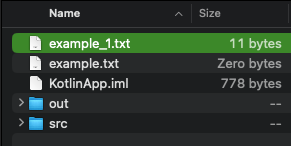
Change the file path and run the program.
Main.kt
import java.io.File
fun main() {
val filePath = "example_1.txt"
val file = File(filePath)
if (file.exists()) {
if (file.length() == 0L) {
println("The file '$filePath' is empty.")
} else {
println("The file '$filePath' is not empty.")
}
} else {
println("The file '$filePath' does not exist.")
}
}
Output
The file 'example_1.txt' is not empty.
Summary
In this Kotlin File Operations tutorial, we have seen how to check if given file is empty or not, using java.io.File.length() method.