Kotlin – Read Text File Line by Line
To read a text file line by line in Kotlin, you can use BufferedReader class from the java.io
package.
In this tutorial, we shall see a step by step guide on how to read a text file line by line in Kotlin, and an example program to read 'example.txt'
line by line.
Steps to read a text file line by line
The following is a step by step process to read a text file line by line, where the text file is given by a filePath.
- Import
BufferedReader
andFileReader
classes from thejava.io
package. - Given a string value in the
filePath
variable with the path to your text file. - Inside the
try
block, create aBufferedReader
by passing aFileReader
that reads from the file specified byfilePath
. - Use a while loop to read the file line by line. The loop continues until there are no more lines to read.
- Inside the loop, you can process each line as needed.
- Finally, close the
BufferedReader
in afinally
block to ensure that the file is properly closed, whether an exception is thrown or not.
Example Program to read ‘example.txt’ line by line
In this example, we have a text file example.txt
which we read the contents line by line.
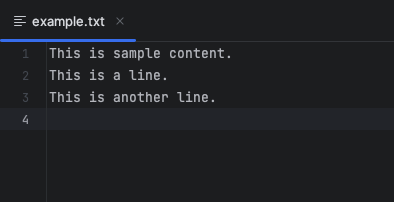
Main.kt
import java.io.BufferedReader
import java.io.FileReader
fun main() {
val filePath = "example.txt" // Replace with the path to your text file
var reader: BufferedReader? = null
try {
reader = BufferedReader(FileReader(filePath))
var line: String?
while (reader.readLine().also { line = it } != null) {
// Process each line
println(line)
}
} catch (e: Exception) {
println("An error occurred: ${e.message}")
} finally {
try {
reader?.close()
} catch (e: Exception) {
println("An error occurred while closing the file: ${e.message}")
}
}
}
Output
This is sample content.
This is a line.
This is another line.
Summary
Summarizing this Kotlin File Operations tutorial, we have seen how to read a text file line by line using BufferedReader class, with a well detailed step by step process, and an example program.