Kotlin – Get File Size
To get the file size in Kotlin, you can use length() method of java.io.File
class.
In this tutorial, we shall see a step by step guide on how to get the size of a file in Kotlin, and an example program to get the size of 'example.txt'
file.
Steps to get the File Size in Kotlin
The following is a step by step process to get the size of a file, where the file is given by a filePath.
- Import
java.io.File
class. - Consider that the file path is given in the
filePath
variable, whose size we would like to find. - Create a
File
object by passing thefilePath
. - Check if the file exists using the
exists()
method of theFile
object. - If the file exists, use the
length()
method to get the size of the file in bytes. - Print the file size.
Example Program to read ‘example.txt’ to a string
In this example, we have a text file example.txt
which we like to get the size of.
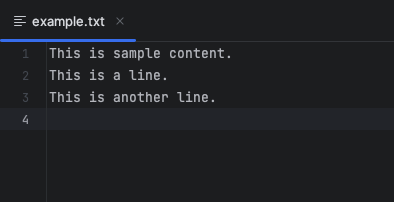
Main.kt
import java.io.File
fun main() {
val filePath = "example.txt" // Replace with the path to your file
val file = File(filePath)
if (file.exists()) {
val fileSize = file.length()
println("File size: $fileSize bytes")
} else {
println("The file '$filePath' does not exist.")
}
}
Output
File size: 62 bytes
Summary
Summarizing this Kotlin File Operations tutorial, we have seen how to get the file size using java.io.File.length()
method, with a well detailed step by step process, and an example program.