Kotlin – Check if a specific String is present in File
To check if a specific string is present in the file in Kotlin, you can read the file content, and then use String contains() method on the file content. The method returns true if the search string is present in the file, or false otherwise. We can use this returned value in an if-else statement to take an action on the result.
Steps to Check if a specific String is present in File
Follow these steps to check if a specific string is present in the file, given by a file path.
- Import
java.io.File
class. - Given file path in
filePath
variable. - Given search string in
searchString
. We need to check if this search string is present in the file. - Create a
File
object using the file pathfilePath
. - Check if the file exists using the
exists()
method. If the file exists, then read the original content of the file usingFile.readText()
to a variable sayfileContents
. - Call String contains() method on the file contents
fileContents
string. The method returns true if searchString is present in the fileContents, otherwise false.
Kotlin Program to Check if Specific String is present in File
In this example program, we shall check if the string "apple"
is present in the file 'example.txt'
.
The original contents of the 'example.txt'
is given below.
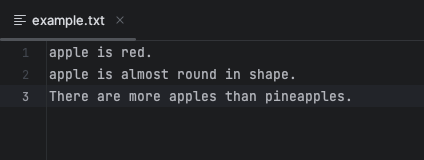
Main.kt
import java.io.File
fun main() {
val filePath = "example.txt" // Replace with the path to your file
val searchString = "apple"
try {
val file = File(filePath)
if (file.exists() && file.isFile) {
val fileContents = file.readText()
if (fileContents.contains(searchString)) {
println("The string '$searchString' is present in the file.")
} else {
println("The string '$searchString' is not present in the file.")
}
} else {
println("File not found or is not a regular file.")
}
} catch (e: Exception) {
println("An error occurred: ${e.message}")
}
}
Output
The string 'apple' is present in the file.
Now, let us take another search string, say "banana"
and check if it is present in the file.
Main.kt
import java.io.File
fun main() {
val filePath = "example.txt" // Replace with the path to your file
val searchString = "banana"
try {
val file = File(filePath)
if (file.exists() && file.isFile) {
val fileContents = file.readText()
if (fileContents.contains(searchString)) {
println("The string '$searchString' is present in the file.")
} else {
println("The string '$searchString' is not present in the file.")
}
} else {
println("File not found or is not a regular file.")
}
} catch (e: Exception) {
println("An error occurred: ${e.message}")
}
}
Output
The string 'banana' is not present in the file.
Since the given string is not present in the file, String contains() method returns false, and the else block executes.
Summary
In this Kotlin File Operations tutorial, we have seen how to check if a specific string in present in the file using File
class and String.contains()
method, with a detailed step by step process, and example programs.