Android TextView – Text Color
To change the text color in TextView widget, set the textColor attribute with required Color value. You can specify color value in rgb
, argb
, rrggbb
, or aarrggbb
formats.
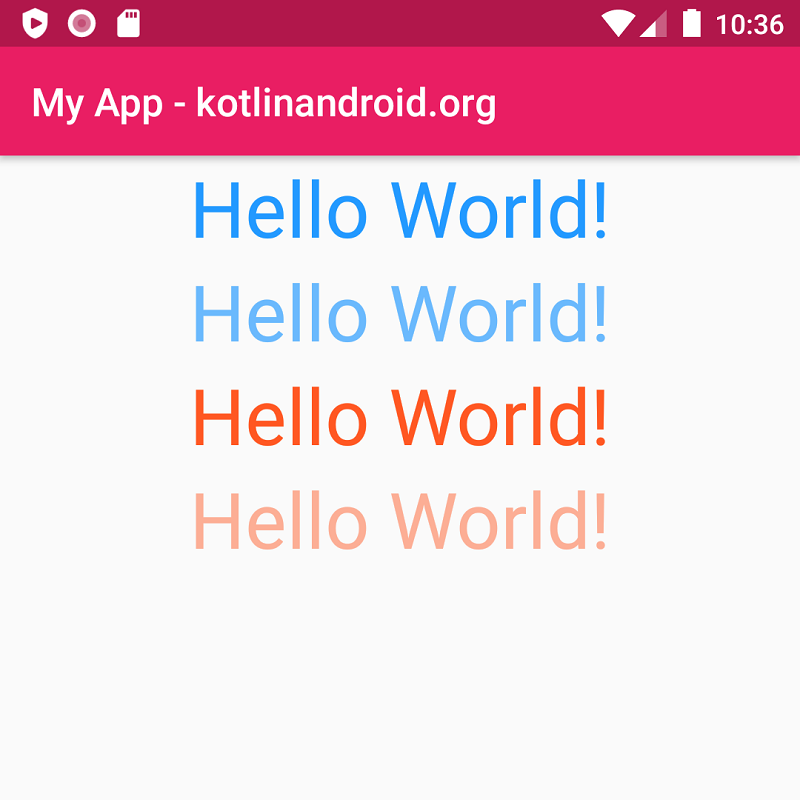
Text Color of TextView in XML Layout
<TextView
android:textColor="#29F"
android:text="Hello World!" />
<TextView
android:textColor="#A29F"
android:text="Hello World!" />
<TextView
android:textColor="#FF5722"
android:text="Hello World!" />
<TextView
android:textColor="#77FF5722"
android:text="Hello World!" />
Example
Create an Android Application with Kotlin support and Empty Activity. Create four TextView widgets with different color formats. The four TextView widgets have the color value in rgb
, argb
, rrggbb
, and aarrggbb
formats respectively.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:textSize="40sp"
android:textColor="#29F"
android:text="Hello World!" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:textSize="40sp"
android:textColor="#A29F"
android:text="Hello World!" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:textSize="40sp"
android:textColor="#FF5722"
android:text="Hello World!" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:textSize="40sp"
android:textColor="#77FF5722"
android:text="Hello World!" />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
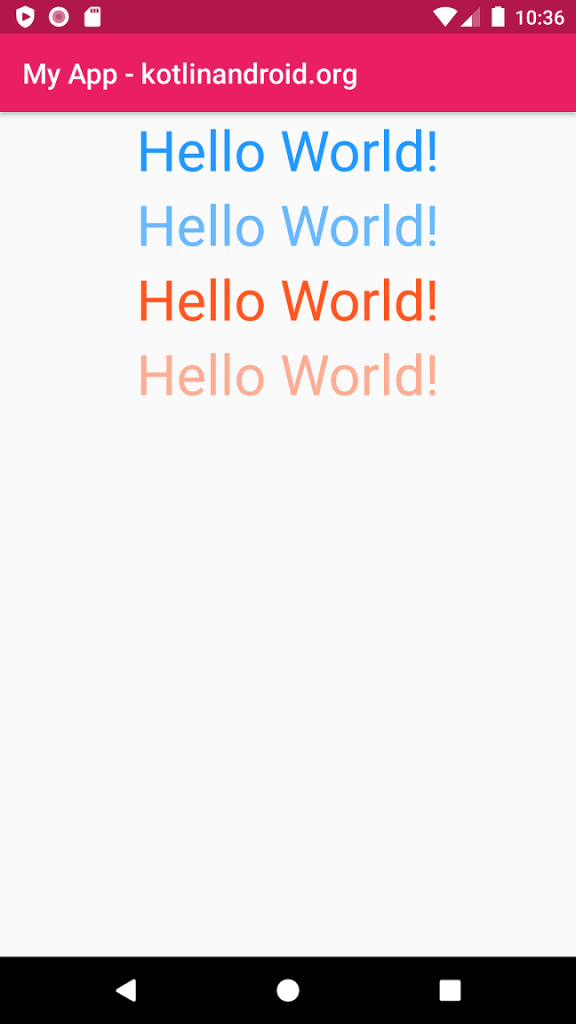