Text Maximum Length in TextView
To set the maximum length for text in TextView widget, set the maxLength attribute with required number of length. If the length of text exceeds the maximum length, then the text is truncated to the specified length.
<TextView
android:maxLength="50"
android:text="Hello World! Welcome to Kotlin Android Tutorial. Learn Android development using Kotlin language." />
<TextView
android:maxLength="60"
android:text="Hello World! Welcome to Kotlin Android Tutorial. Learn Android development using Kotlin language." />
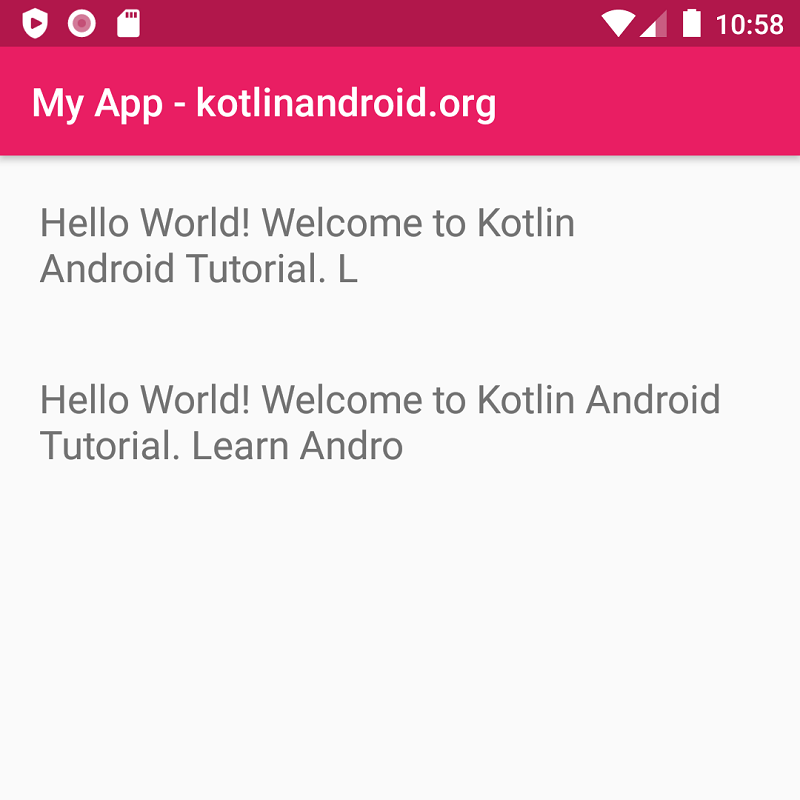
Example
Create an Android Application with Kotlin support and Empty Activity. Create three TextView widgets with different text sizes.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="20dp"
android:textSize="20sp"
android:maxLength="50"
android:text="Hello World! Welcome to Kotlin Android Tutorial. Learn Android development using Kotlin language." />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="20dp"
android:textSize="20sp"
android:maxLength="60"
android:text="Hello World! Welcome to Kotlin Android Tutorial. Learn Android development using Kotlin language." />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
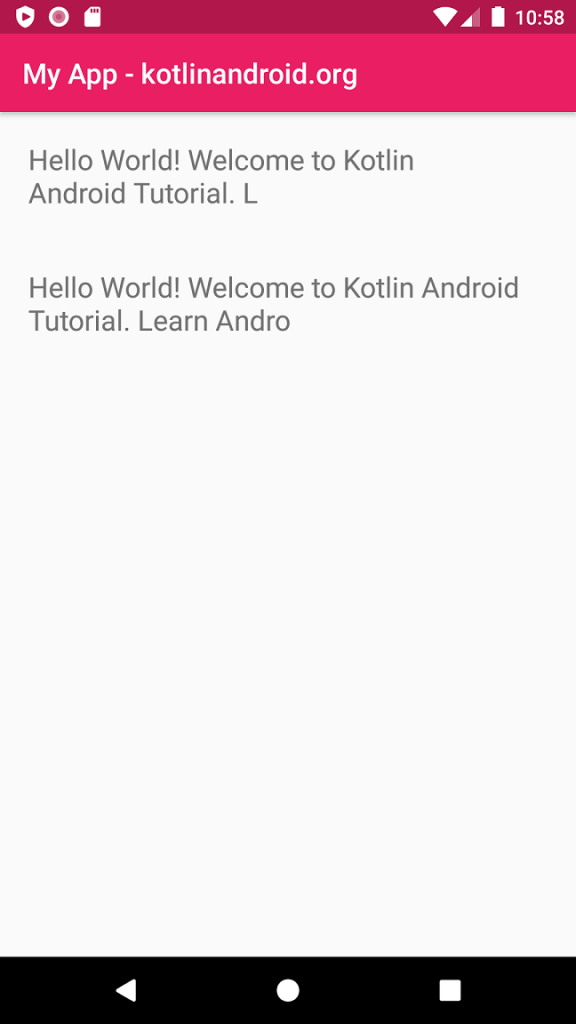