TextView Background Color
To change the background color of TextView widget, set the background attribute with required Color value. You can specify color in rgb
, argb
, rrggbb
, or aarrggbb
formats.
<TextView
android:background="#269"
android:text="Hello World" />
<TextView
android:background="#E3AA"
android:text="Hello World" />
<TextView
android:background="#F6D55C"
android:text="Hello World" />
<TextView
android:background="#DDED553B"
android:text="Hello World" />

The background color is applied along the width and height of the TextView.
In the above screenshot, width and height of the TextViews are modified using padding, margin, width, etc., and different background colors are applied.
Example
Create an Android Application with Kotlin support and Empty Activity. Create four TextView widgets with different background colors covering different Color formats.
The four TextView widgets have the color value in rgb
, argb
, rrggbb
, and aarrggbb
formats respectively.
Also, their dimensions are modified so that we understand which area of the TextView is being painted with the background color.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:background="#269"
android:text="Hello World" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:background="#E3AA"
android:text="Hello World" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="20dp"
android:textSize="30sp"
android:textAlignment="center"
android:background="#F6D55C"
android:text="Hello World" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:background="#DDED553B"
android:text="Hello World" />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
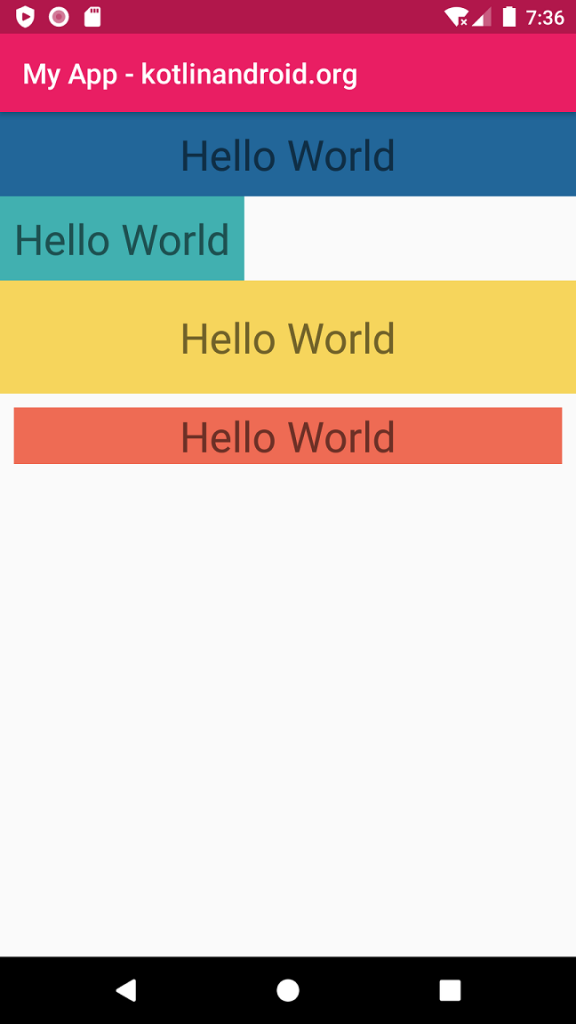