Android Button – setOnClickListener
Android Button setOnClickListener() function is used to execute code when user clicks or taps on the button.
Steps to call setOnClickListener() on Button
To execute a set of statements when user taps on Button widget, follow these steps.
- Get reference to the Button.
- Call setOnClickListener() on the Button reference. You can use lambda expression with setOnClickListener() function.
- Write the set of statements inside setOnClickListener() function block. This code block gets executed when a tap happens on the button.
The typical syntax to call setOnClickListener() on a Button is
val button = findViewById<Button>(R.id.button_id)
button.setOnClickListener {
//cdoe that executes when user taps on the button
}
Example
Create an Android Application with Kotlin support and Empty Activity. Create a Button widget with id specified and text value taken from strings.xml.
strings.xml
<resources>
<string name="app_name">My App - kotlinandroid.org</string>
<string name="submit">Submit</string>
</resources>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/button_id"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:layout_margin="10dp"
android:paddingHorizontal="40dp"
android:textAllCaps="false"
android:background="#FFF"
android:textSize="25sp"
android:text="@string/submit" />
</LinearLayout>
In the kotlin file, we will set the Button’s setOnClickListener() function in MainActivity’s onCreate() function. We shall set the content view for the activity with the layout file, and then set the action listener for button.
When user clicks or taps on the button, we are just showing a Toast. You may replace this with your own code as required.
MainActivity.kt
package com.kotlinandroid.myapp
import android.os.Bundle
import android.widget.Button
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button_id)
button.setOnClickListener {
Toast.makeText(applicationContext,"You clicked Submit Button.",Toast.LENGTH_SHORT).show()
}
}
}
Screenshot
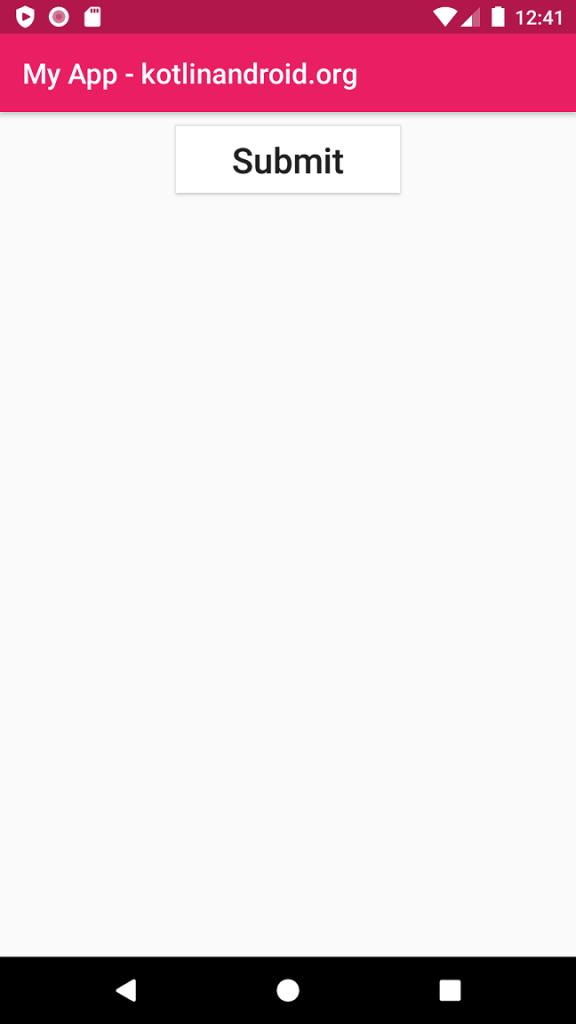
Click on the button. You will see a Toast made in the bottom area of the screen.
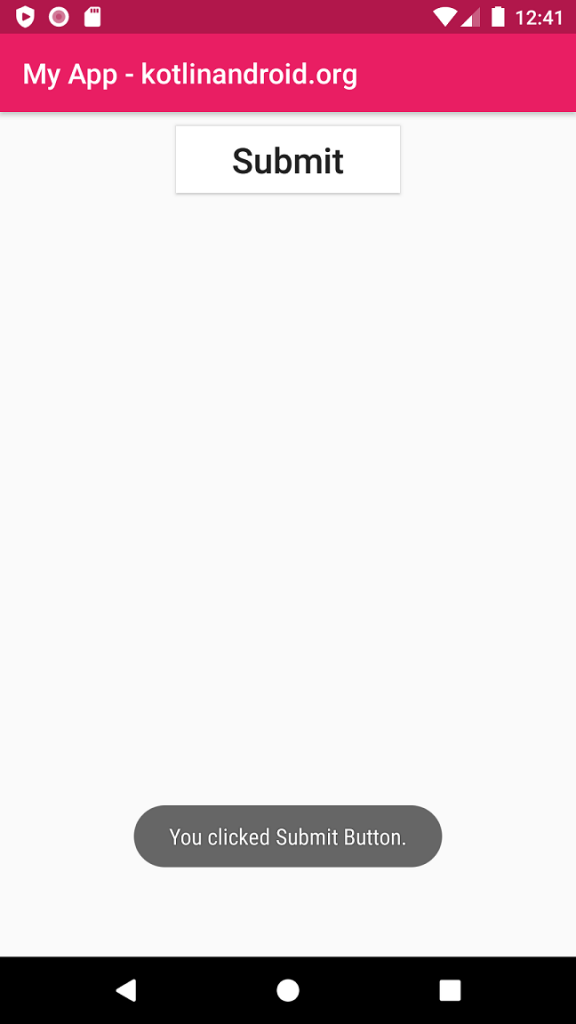