Center Align Text in TextView
To center align text in TextView widget, set the textAlignment attribute of the widget to center
value.
The default text alignment of text in TextView is left.
Note: When you specify text alignment, it takes effect only when the width of TextView widget is more than the width of text. In other words, the size of container should be greater than the size of content, or, if the text spans multiple lines.
<TextView
android:textAlignment="center"
android:text="Center Aligned Text" />
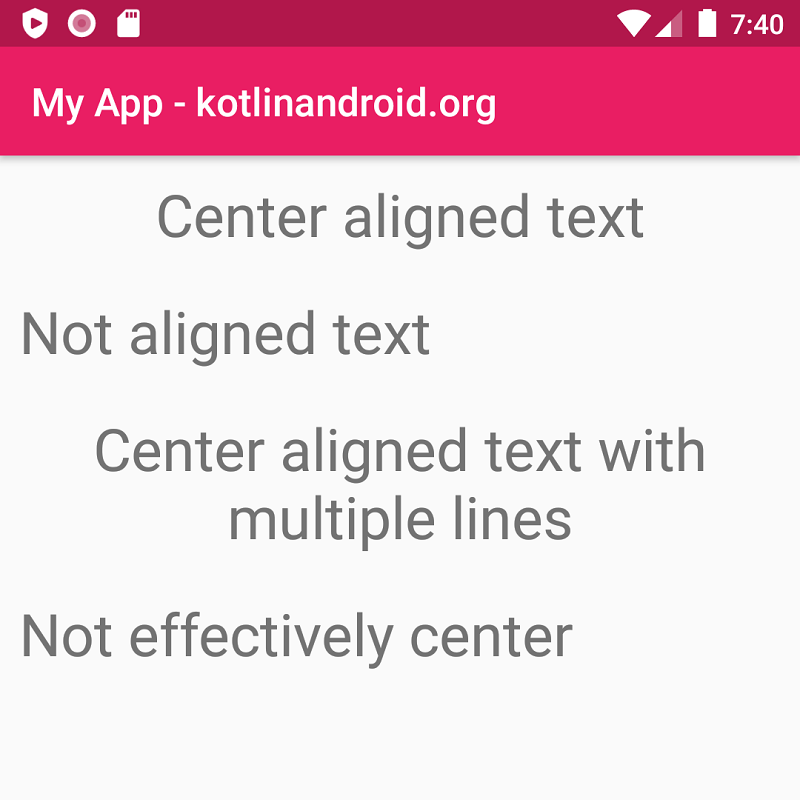
Example
Create an Android Application with Kotlin support and Empty Activity. Create four TextView widgets with different values for layout width and text alignment.
- First TextView – Width of the widget matches the parent, which is the LinearLayout, and of course the width of the device. TextView width is greater than the width of text. So, effectively displayed with center alignment.
- Second TextView – Default text alignment which is left. Width of TextView or its text does not affect.
- Third TextView – Width of the widget wraps the content, which is the text. But as text spans multiple lines, the text is effectively displayed with center alignment.
- Forth TextView – Width of the widget wraps the content, which is the text. But as width of TextView is not greater than the width of text, not the text is spanned across multiple lines, the text is effectively displayed with default alignment.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:text="Center aligned text" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:text="Not aligned text" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:text="Center aligned text with multiple lines" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:textSize="30sp"
android:textAlignment="center"
android:text="Not effectively center" />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
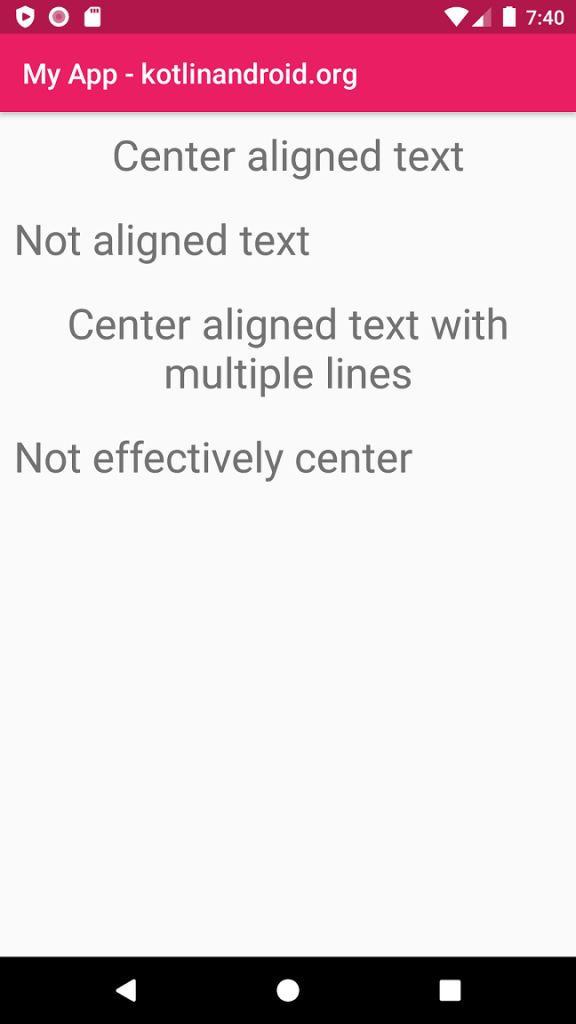