Android Button Widget
Android Button widget is an User Interface elements that allows user to tap or click to perform an action.
Button XML
Code snippet for a simple Android Button widget in XML layout file is
<Button
android:id="@+id/button_id"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:text="@string/submit" />
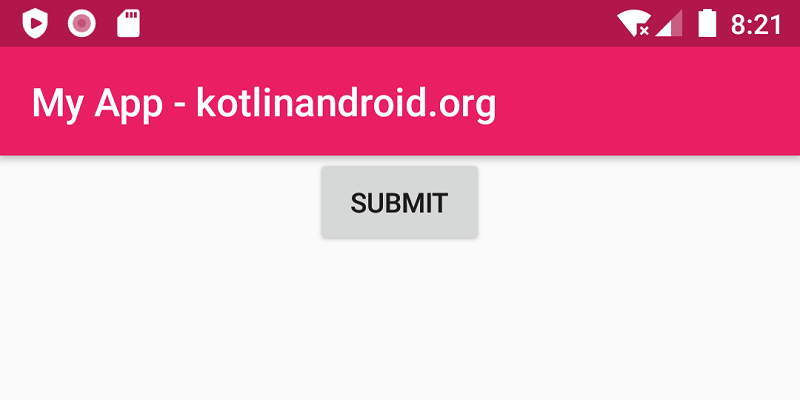
You can set many attributes supported by Android Button widget to change its appearance and behavior.
Some of the attributes that change the look of Android Button is given in the following example Button code.
<Button
android:id="@+id/button_id"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:layout_margin="10dp"
android:paddingHorizontal="40dp"
android:textAllCaps="false"
android:textSize="20sp"
android:background="#0789C5"
android:textColor="#FFF"
android:text="@string/submit" />
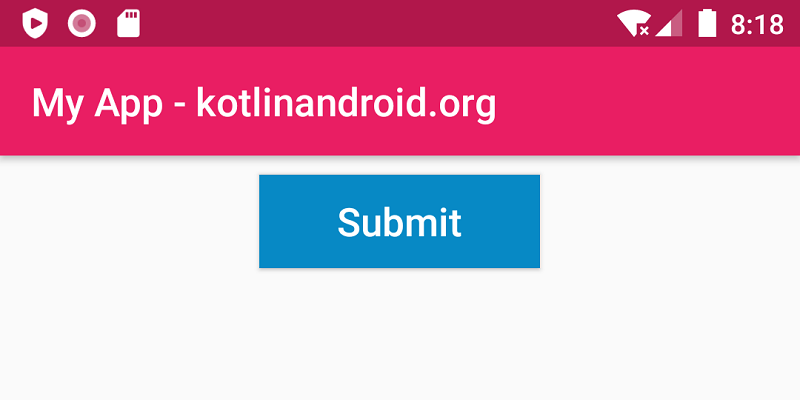
Example
Create an Android Application with Kotlin support and Empty Activity. Create a Button widget with some of its attributes set for a modified look.
strings.xml
<resources>
<string name="app_name">My App - kotlinandroid.org</string>
<string name="submit">Submit</string>
</resources>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/button_id"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:layout_margin="10dp"
android:paddingHorizontal="40dp"
android:textAllCaps="false"
android:textSize="20sp"
android:background="#0789C5"
android:textColor="#FFF"
android:text="@string/submit" />
</LinearLayout>
MainActivity.kt
package com.kotlinandroid.myapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Screenshot
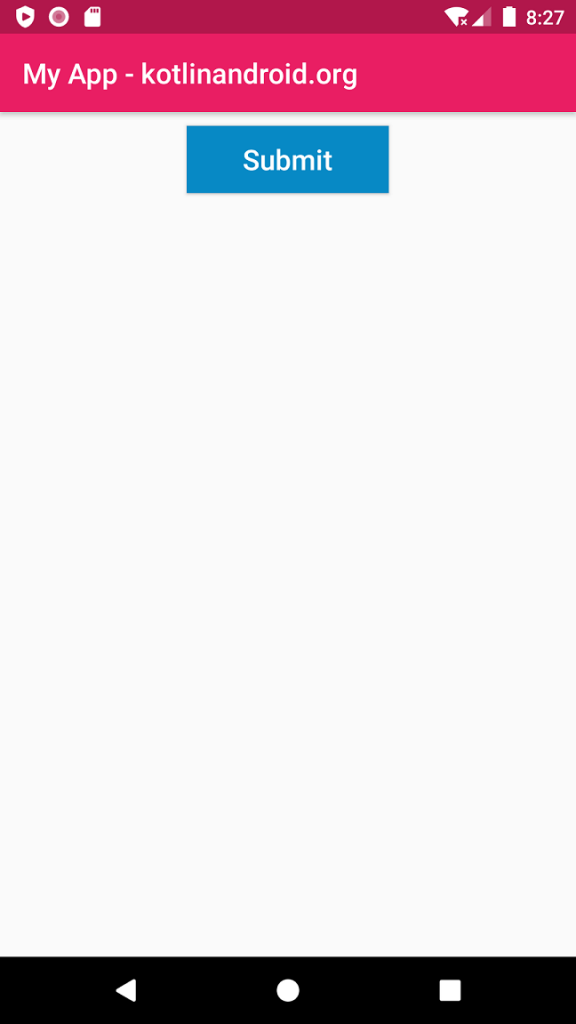
If you tap on the button, nothing happens. This is because, we have not set any action listener for the button.
Let us go ahead and set an action listener for the Android Button, such that it displays a Toast when user taps on the Button.
All the code remains same, except for the MainActivity.kt.
MainActivity.kt
package com.kotlinandroid.myapp
import android.os.Bundle
import android.widget.Button
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button_id)
button.setOnClickListener {
Toast.makeText(applicationContext,"You clicked Submit Button.",Toast.LENGTH_SHORT).show()
}
}
}
Screenshot
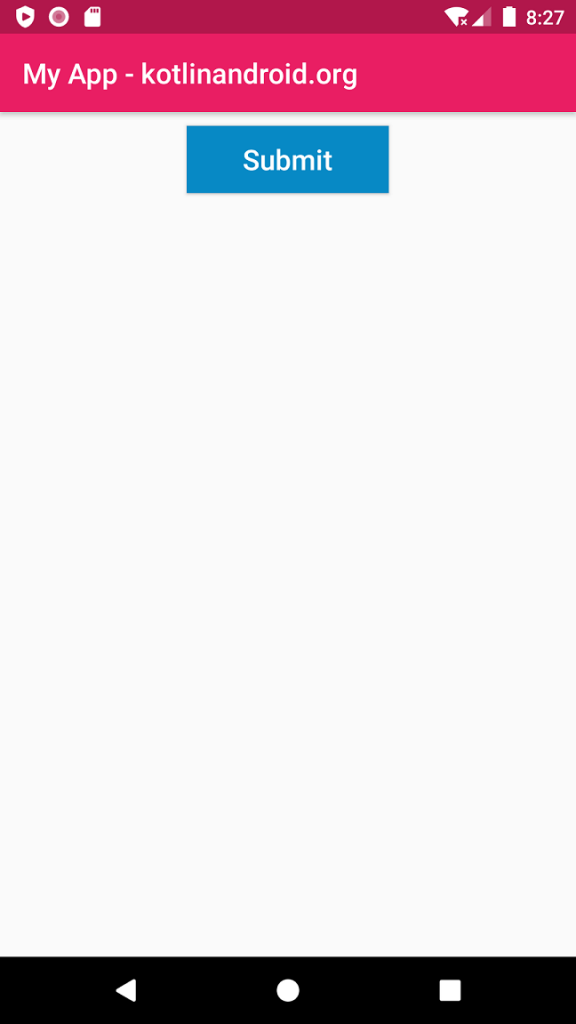
Click on the Submit button.
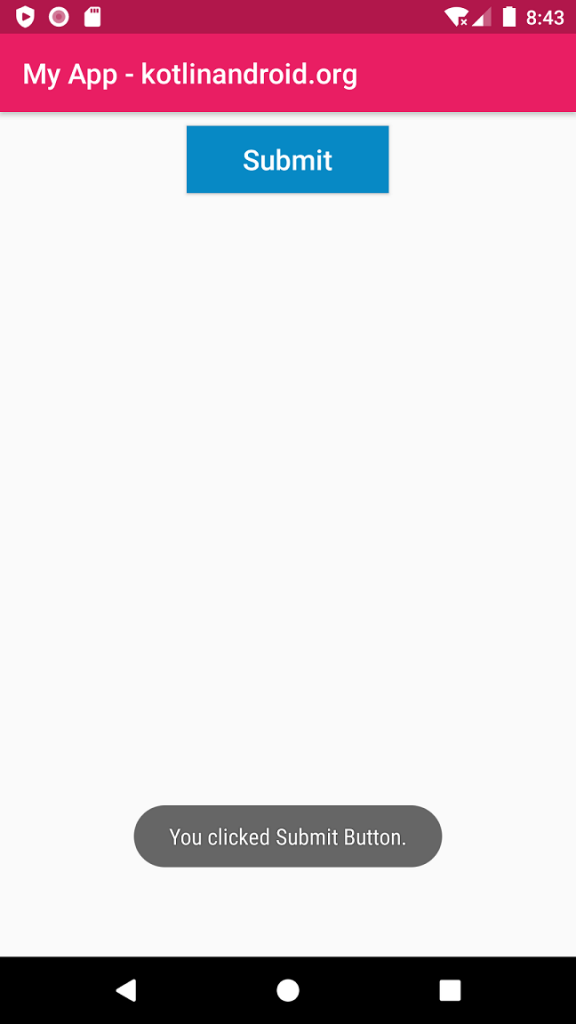