Kotlin – Single-Line Comments
In Kotlin, you can add single-line comments to your code to provide explanations, notes, or reminders. Single-line comments are ignored by the compiler and are used for documentation or to annotate specific lines of code.
To add a single-line comment in Kotlin, you can use the //
syntax. Any text following //
on the same line is treated as a comment and is not executed by the compiler.
// This is a single-line comment
val number = 42 // Assigning value to a variable
In the example above, // This is a single-line comment
is a single-line comment that provides a description, and // Assigning value to a variable
is a comment annotating the code that follows it.
Single-line comments are useful for clarifying code, explaining the purpose of variables or functions, or temporarily disabling code without deleting it.
Examples
1. Adding Comments to Code
In the following example, we use single-line comments to explain each line of code.
Kotlin Program
fun main() {
// Define a variable
val message = "Hello, Kotlin!"
// Print the message
println(message)
// Print hello world
println("Hello World")
}
In IDE
You can easily differentiate between the comments and actual code in an IDE. The following is an example screenshot in IntelliJ IDEA.
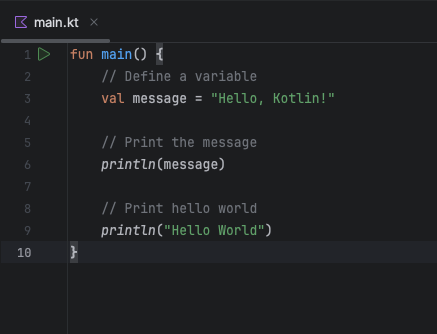
2. Temporarily Disabling Code
You can also use single-line comments to temporarily disable lines of code without deleting them.
Kotlin Program
// Define a variable
val number = 42
// Print the number
println(number)
// Disable the following line temporarily
// println("This line is commented out")
In this example, the line println("This line is temporarily disabled")
is commented out and won’t be executed until the comment is removed.
In IDE
You can easily differentiate between the comments and actual code in an IDE. The following is an example screenshot in IntelliJ IDEA.
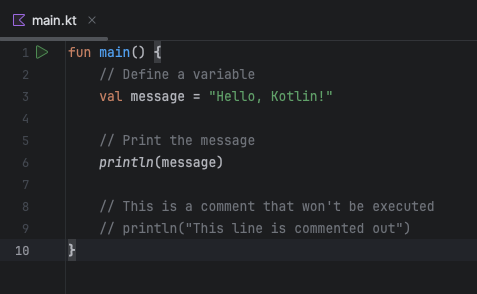
Summary
Single-line comments in Kotlin are created using the //
syntax. They are used to add explanations, notes, or annotations to your code. Single-line comments are ignored by the compiler and are helpful for documentation and code organization.