How to Read Integer from Console in Kotlin?
To read an integer from the console entered by the user in Kotlin, you can use the readLine()
function along with toInt()
conversion.
val input = readLine()?.toInt()
When the readLine()
function is executed, a prompt appears in the standard console input, and the user can enter an input there. After entering the input in the console, press the enter key. The entered input is returned as a string by the readLine()
function.
Examples
1. Read an integer from console and print its square value
In the following program, we read an integer from the console, and if the input is not null, we perform operations on it.
Kotlin Program
fun main() {
print("Enter an integer: ")
val inputInteger = readLine()?.toInt()
if (inputInteger != null) {
val squaredValue = inputInteger * inputInteger
println("Square of $inputInteger is $squaredValue")
} else {
println("Invalid input. Please enter a valid integer.")
}
}
Output
The following is the sample output when user enters an integer value in the console input.
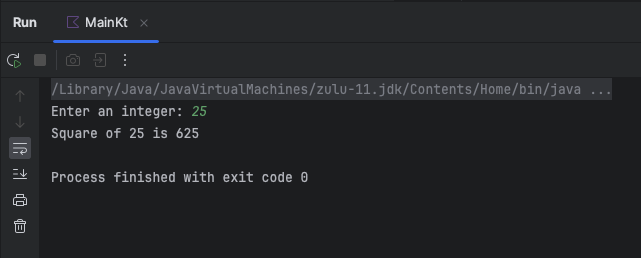
The following is the sample output when user enters an invalid integer value like some alphabet characters in the console input.
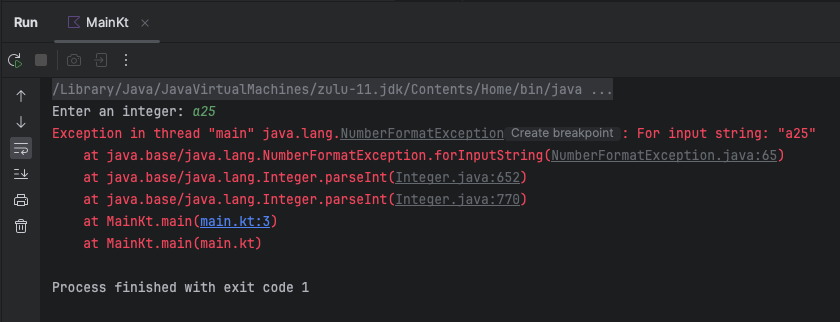
Program Explanation
- User Input Prompt:
print("Enter an integer: ")
: This line prints the message “Enter an integer: ” to the console, prompting the user to enter an integer.
- Reading User Input and Conversion:
val inputInteger = readLine()?.toInt()
: This line reads a line of input from the user through the console. ThereadLine()
function returns a string, which is then converted to an integer using thetoInt()
function. The?
is for safe call, which means if the input is null, it won’t throw an exception but instead return null.
- Checking Input and Performing Calculation:
if (inputInteger != null) { ... }
: This line checks if the input stored ininputInteger
is not null. If the user entered a valid integer, this block of code will execute.val squaredValue = inputInteger * inputInteger
: Inside the previous if block, this line calculates the square of the input integer by multiplying it with itself.println("Square of $inputInteger is $squaredValue")
: If the inputinputInteger
is valid, this line prints a message to the console indicating the square of the input integer.
- Handling Invalid Input:
else { ... }
: If the inputinputInteger
is null (i.e., the user did not enter a valid integer), this block of code will execute.println("Invalid input. Please enter a valid integer.")
: This line prints a message to the console indicating that the input was invalid and prompts the user to enter a valid integer.
- Complete Program Structure:
- The entire program is wrapped within the
main()
function, which is the entry point of a Kotlin program. - The program prompts the user to enter an integer, reads the input, converts it to an integer, checks if it’s valid, calculates the square if valid, and handles invalid input.
- The entire program is wrapped within the