Kotlin Multiline Comments
In Kotlin, multiline comments are used to write longer explanations, comments, or annotations that span multiple lines. These comments are ignored by the compiler and are typically used for documentation purposes or to temporarily disable large blocks of code.
Adding Multiline Comments in Kotlin Code
To add a multiline comment in Kotlin, you can use the /* */
syntax. Any text enclosed between /*
and */
is treated as a comment and is not executed by the compiler.
/*
This is a multiline comment
that spans multiple lines.
It can contain explanations, notes,
or annotations.
*/
Unlike single-line comments, multiline comments can span across several lines and can include line breaks, making them suitable for longer comments or documentation blocks.
Kotlin Program
fun main() {
// Define a variable
val message = "Hello, Kotlin!"
// Print the message
println(message)
/*
This is a multiline comment
that spans multiple lines.
It can contain explanations, notes,
or annotations.
*/
}
Display of multiline comments in IDE
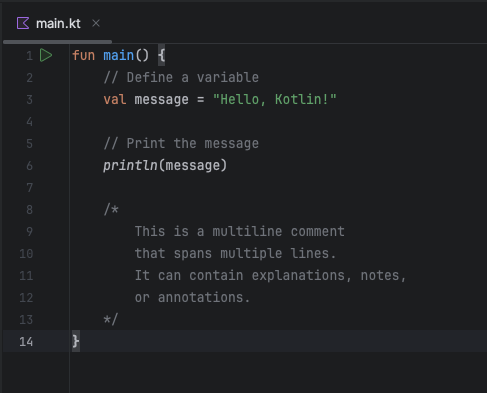
Code in Multiline Comments
In the following example, we use a multiline comment to comment out a whole function.
fun main() {
// Define a variable
val message = "Hello, Kotlin!"
// Print the message
println(message)
}
/*
fun calculateSum(a: Int, b: Int): Int {
// This function calculates the sum of two integers
return a + b
}
*/
Display of multiline comments in IDE

Summary
Kotlin multiline comments are enclosed between /*
and */
and can span multiple lines. They are useful for writing detailed explanations, comments, or annotations in your code.