Kotlin List take()
The List take()
function in Kotlin is used to create a new list containing the first n elements from the original list. It returns a new list with the specified number of elements.
Syntax
The take()
function has the following syntax:
fun <T> List<T>.take(n: Int): List<T>
Parameters
Parameter | Description |
---|---|
n | The number of elements to take from the beginning of the list. |
Return Value
The take()
function returns a new list containing the first n elements from the original list. If the original list has fewer than n elements, it returns the entire original list.
Example 1: Taking Elements from a List
This example demonstrates how to use take()
to create a new list with the first n elements from the original list.
In this example,
- Create a list of numbers, numbers.
- Use
take()
to create a new list containing the first 3 elements from the list. - The
take()
function returns a new list with elements[1, 2, 3]
.
Kotlin Program
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val firstThree = numbers.take(3)
println("First three elements: $firstThree")
}
Output
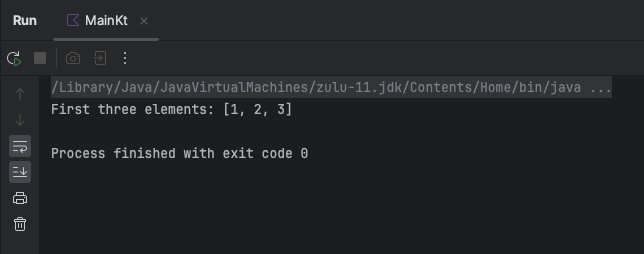
Example 2: Taking Elements from an Empty List
In this example, we use take()
to create a new list from an empty list.
In this example,
- Create an empty list, emptyList.
- Use
take()
to create a new list containing the first 3 elements from emptyList. - The
take()
function returns an empty list because the original list is empty.
Kotlin Program
fun main() {
val emptyList = emptyList<Int>()
val firstThree = emptyList.take(3)
println("First three elements from empty list: $firstThree")
}
Output
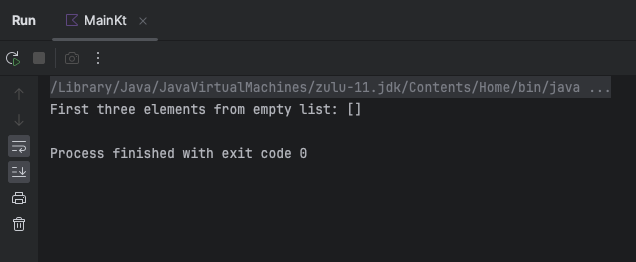
Summary
The take()
function in Kotlin is useful for creating a new list containing the first n elements from the original list. It handles cases where the original list has fewer than n elements by returning the entire original list.