Kotlin List shuffled()
The shuffled()
function in Kotlin is used to shuffle the elements of a list and return a new list with the elements rearranged randomly.
Syntax
The shuffled()
function takes a Random
object as input and returns a new list with the elements shuffled randomly.
fun <T> Iterable<T>.shuffled(random: Random): List<T>
Parameter | Description |
---|---|
random | A Random object used for shuffling the elements. |
Return Value
The shuffled()
function returns a new list with the elements of the original list shuffled randomly.
Example 1: Basic Usage
This example demonstrates how to use shuffled()
to shuffle the elements of a list.
In this example,
- Create a list of numbers in the variable numbers.
- Call
shuffled()
function on the list numbers with aRandom
object. - The
shuffled()
function returns a new list with the elements of numbers shuffled randomly.
Kotlin Program
import kotlin.random.Random
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val shuffledList = numbers.shuffled(Random)
println(shuffledList) // Output: Randomly shuffled list
}
Output
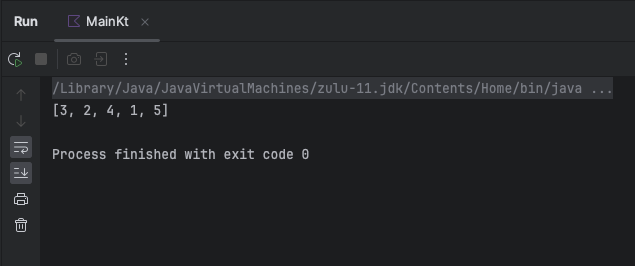
Example 2: Shuffling a List of Strings
In this example, we shuffle a list of strings using shuffled()
.
In this example,
- Create a list of strings in the variable colors.
- Call
shuffled()
function on the list colors with aRandom
object. - The
shuffled()
function returns a new list with the elements of colors shuffled randomly.
Kotlin Program
import kotlin.random.Random
fun main() {
val colors = listOf("Red", "Green", "Blue", "Yellow")
val shuffledColors = colors.shuffled(Random)
println(shuffledColors) // Output: Randomly shuffled list of colors
}
Output
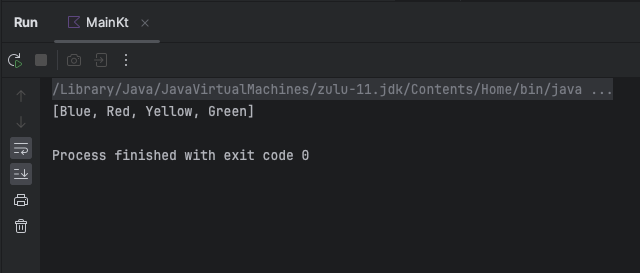
Summary
The shuffled()
function in Kotlin is useful for randomly shuffling the elements of a list. We have seen the syntax and basic usage of the shuffled()
function with examples.