Kotlin List random()
The random()
function in Kotlin is used to get a random element from a list. It returns a randomly selected element from the list.
Syntax
The random()
function has the following syntax:
fun <T> List<T>.random(): T
Return Value
The random()
function returns a randomly selected element from the list. If the list is empty, it throws NoSuchElementException
.
Example 1: Getting a Random Number
This example demonstrates how to use random()
to get a random number from a list.
In this example,
- Create a list of numbers, numbers.
- Use
random()
to get a random number from the list. - The
random()
function returns a randomly selected number from the list.
Kotlin Program
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val randomNum = numbers.random()
println("Random number: $randomNum")
}
Output
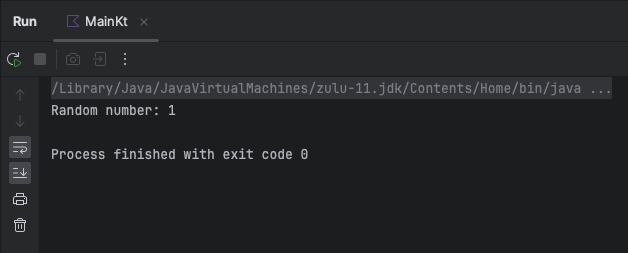
Example 2: Getting a Random String
In this example, we use random()
to get a random string from a list.
In this example,
- Create a list of strings, words.
- Use
random()
to get a random string from the list. - The
random()
function returns a randomly selected string from the list.
Kotlin Program
fun main() {
val words = listOf("apple", "banana", "cherry", "orange")
val randomWord = words.random()
println("Random word: $randomWord")
}
Output
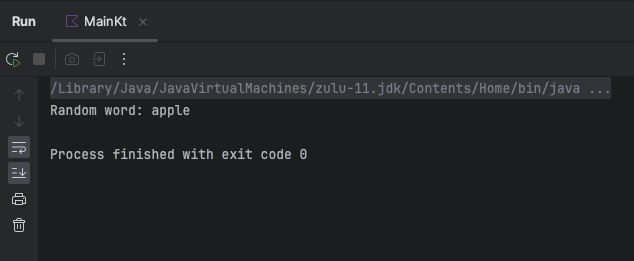
Summary
The random()
function in Kotlin is useful for getting a random element from a list. It can be used with lists of numbers, strings, or any other type of element. In this tutorial, we have learned the syntax of List.random() function, and how to use this function with the help of Kotlin examples.