Kotlin List onEach()
The onEach()
function in Kotlin is used to perform an action on each element of the list without changing the original list. It applies the specified operation to each element and returns the same list.
Syntax
The onEach()
function has the following syntax:
fun <T> Iterable<T>.onEach(
action: (T) -> Unit
): Iterable<T>
Parameters
Parameter | Description |
---|---|
action | A function that takes an element of type T and performs some action on it. |
Return Value
The onEach()
function returns the same list after applying the specified action to each element.
Example 1: Printing Each Element
This example demonstrates how to use onEach()
to print each element of a list.
In this example,
- Create a list of numbers, numbers.
- Use
onEach()
to print each number in the list. - The
onEach()
function applies theprintln()
action to each number in the list. - The original list remains unchanged.
Kotlin Program
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
numbers.onEach { println(it) }
}
Output
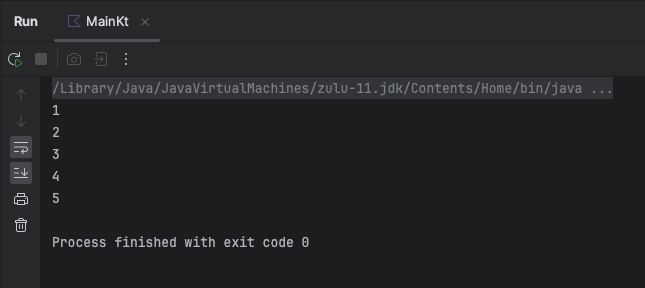
Example 2: Mapping Elements
In this example, we use onEach()
to map each element of a list to its squared value.
In this example,
- Create a list of numbers, numbers.
- Use
onEach()
to square each number in the list. - The
onEach()
function applies themap()
action to each number in the list, transforming it to its squared value. - The original list remains unchanged.
Kotlin Program
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
numbers.onEach { println(it * it) }
}
Output
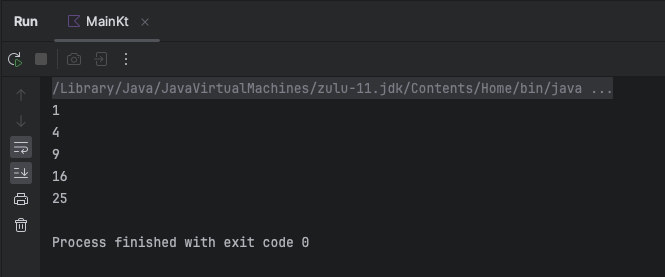
Summary
The onEach()
function in Kotlin is useful for performing actions on each element of a list without modifying the original list. It can be used for printing elements, mapping elements, or any other action that needs to be performed on each element.
We have seen the syntax, and basic usage of the List.onEach() function with a couple of examples.