Kotlin List indexOfLast()
The indexOfLast()
function in Kotlin is used to find the index of the last element in a list that matches a given predicate.
Syntax
The indexOfLast()
function takes a predicate as input and returns the index of the last element in the list that satisfies the predicate. If no element matches the predicate, it returns -1.
fun <T> List<T>.indexOfLast(predicate: (T) -> Boolean): Int
Parameters
Parameter | Description |
---|---|
predicate | A function that takes an element of type T and returns true if the element matches the criteria, false otherwise. |
Return Value
The indexOfLast()
function returns the index of the last element that matches the predicate. If no element matches, it returns -1.
Example 1: Finding the Index of Last Even Number
This example demonstrates how to use indexOfLast()
to find the index of the last even number in a list.
In this example,
- Create a list of numbers in the variable numbers.
- Define a predicate function that checks if a number is even.
- Call
indexOfLast()
on the list numbers with the predicate function. - The
indexOfLast()
function returns the index of the last even number in the list.
Kotlin Program
fun main() {
val numbers = listOf(1, 3, 5, 2, 4, 6)
val index = numbers.indexOfLast { it % 2 == 0 }
println("Index of last even number: $index")
}
Output
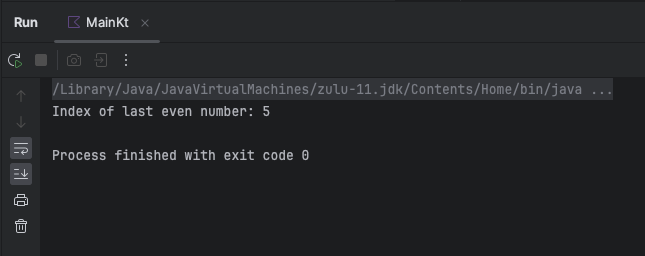
Example 2: Finding Index of Last String Starting with “A”
In this example, we find the index of the last string in a list that starts with the letter “A”.
In this example,
- Create a list of strings in the variable names.
- Define a predicate function that checks if a string starts with the letter “A”.
- Call
indexOfLast()
on the list names with the predicate function. - The
indexOfLast()
function returns the index of the last string starting with “A” in the list.
Kotlin Program
fun main() {
val names = listOf("Alice", "Bob", "Anna", "John")
val index = names.indexOfLast { it.startsWith("A") }
println("Index of last name starting with 'A': $index")
}
Output
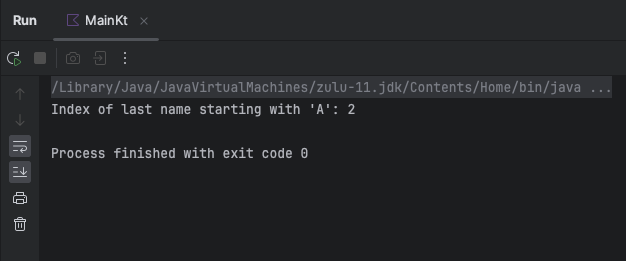
Summary
The indexOfLast()
function in Kotlin is useful for finding the index of the last element in a list that matches a given predicate. We have seen examples of using it with different criteria.