Kotlin List indexOfFirst()
The indexOfFirst()
function in Kotlin is used to find the index of the first element in a list that matches a given predicate.
Syntax
The indexOfFirst()
function takes a predicate as input and returns the index of the first element in the list that satisfies the predicate. If no element matches the predicate, it returns -1.
fun <T> List<T>.indexOfFirst(predicate: (T) -> Boolean): Int
Parameters
Parameter | Description |
---|---|
predicate | A function that takes an element of type T and returns true if the element matches the criteria, false otherwise. |
Return Value
The indexOfFirst()
function returns the index of the first element that matches the predicate. If no element matches, it returns -1.
Example 1: Finding the Index of First Even Number
This example demonstrates how to use indexOfFirst()
to find the index of the first even number in a list.
In this example,
- Create a list of numbers in the variable numbers.
- Define a predicate function that checks if a number is even.
- Call
indexOfFirst()
on the list numbers with the predicate function. - The
indexOfFirst()
function returns the index of the first even number in the list.
Kotlin Program
fun main() {
val numbers = listOf(1, 3, 5, 2, 4, 6)
val index = numbers.indexOfFirst { it % 2 == 0 }
println("Index of first even number: $index")
}
Output
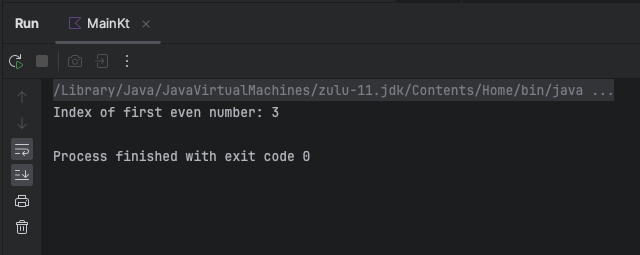
Example 2: Finding Index of First String Starting with “A”
In this example, we find the index of the first string in a list that starts with the letter “A”.
In this example,
- Create a list of strings in the variable names.
- Define a predicate function that checks if a string starts with the letter “A”.
- Call
indexOfFirst()
on the list names with the predicate function. - The
indexOfFirst()
function returns the index of the first string starting with “A” in the list.
Kotlin Program
fun main() {
val names = listOf("Alice", "Bob", "Anna", "John")
val index = names.indexOfFirst { it.startsWith("A") }
println("Index of first name starting with 'A': $index")
}
Output
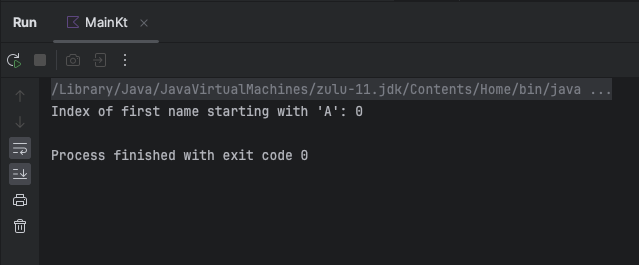
Summary
The indexOfFirst()
function in Kotlin is useful for finding the index of the first element in a list that matches a given predicate. We have seen examples of using it with different criteria.