Examples for If statement in Kotlin
In this tutorial, we will go through some examples for If statement in Kotlin programming language.
Example 1: Check if given number is exactly divisible by 10
In the following program, we read an integer from console, and check if the integer is exactly divisible by 10, using an if-statement.
Kotlin Program
fun main() {
print("Enter an integer : ")
val x = readLine()?.toInt()
if (x != null) {
if (x % 10 == 0) {
println("$x is exactly divisible by 10.")
}
}
}
Output#1
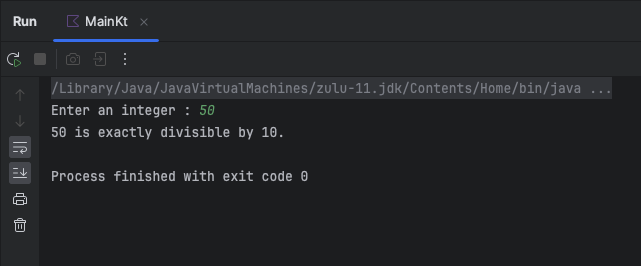
Output#2
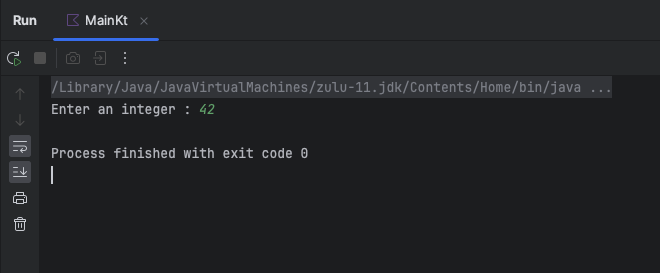
Program Explanation
- User Input Prompt:
print("Enter an integer : ")
: This line prints the message “Enter an integer : ” to the console.
- Reading User Input:
val x = readLine()?.toInt()
: This line reads a line of input from the user through the console. ThereadLine()
function returns a string, and?.toInt()
attempts to convert that string to an integer. The?
is for safe call, which means if the input is null, it won’t throw an exception but instead return null.
- Checking Input and Performing Calculation:
if (x != null) { ... }
: This line checks if the inputx
is not null. If the user entered a valid integer, this block of code will execute.if (x % 10 == 0) { ... }
: Inside the previous if block, this line checks if the inputx
is exactly divisible by 10. The expressionx % 10 == 0
checks if the remainder ofx
divided by 10 is equal to 0, which meansx
is divisible by 10 without any remainder.println("$x is exactly divisible by 10.")
: If the inputx
is divisible by 10, this line prints a message to the console indicating that fact. The$x
is a string template that inserts the value ofx
into the string.
- Complete Program Structure:
- The entire program is wrapped within the
main()
function, which is the entry point of a Kotlin program. - The program prompts the user to enter an integer, reads the input, checks if it’s divisible by 10, and prints a message if it is.
- The entire program is wrapped within the
Example 2: Check if given string is “apple”
In the following program, we read an string from console, and check if the given string is equal to "apple"
, using an if-statement.
Kotlin Program
fun main() {
print("Enter correct name : ")
val x = readLine()
if (x == "apple") {
println("Given fruit is correct.")
}
}
Output#1
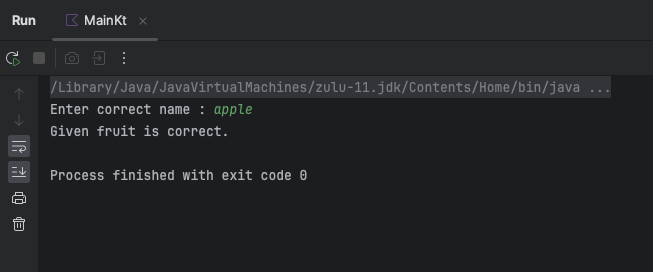
Output#2
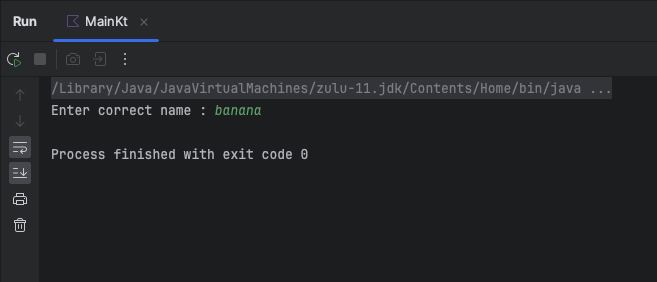
Program Explanation
- User Input Prompt:
print("Enter correct name : ")
: This line prints the message “Enter correct name : ” to the console, prompting the user to enter a name.
- Reading User Input:
val x = readLine()
: This line reads a line of input from the user through the console. ThereadLine()
function returns a string, which is stored in the variablex
.
- Checking Input and Printing Message:
if (x == "apple") { ... }
: This line checks if the input stored inx
is equal to the string “apple.”println("Given fruit is correct.")
: If the inputx
is equal to “apple,” this line prints a message to the console indicating that the given name is correct.
- Complete Program Structure:
- The entire program is wrapped within the
main()
function, which is the entry point of a Kotlin program. - The program prompts the user to enter a correct name, reads the input, checks if it’s equal to “apple,” and prints a message if it is.
- The entire program is wrapped within the