Switch unchecked thumb content in Android Jetpack Compose
We set a specific content in thumb of a Switch
composable, when the switch is unchecked.

To set specific thumb content when the switch is unchecked in Android Jetpack Compose, you can assign a composable to thumbContent
parameter of the Switch
composable, based on the condition that the switch is unchecked.
For example, in the following Switch
composable, we set the thumb content with a “Icons.Filled.Lock” icon when the switch is unchecked.
var checked by remember { mutableStateOf(false) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = if (checked) {
// thumb content when switch is checked
null
} else {
{
Icon(
imageVector = Icons.Filled.Lock,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
}
)

Let us use other icons like “Icons.Filled.Clear” for thumb content when switch is unchecked.
var checked by remember { mutableStateOf(false) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = if (checked) {
// thumb content when switch is checked
null
} else {
{
Icon(
imageVector = Icons.Default.Clear,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
}
)

Example Android Application for Switch thumb content when unchecked
Now, let us create an Android application with Switch
composable, where we set the thumb content of the switch when it is unchecked.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Favorite
import androidx.compose.material.icons.filled.Lock
import androidx.compose.material.icons.filled.Warning
import androidx.compose.material3.Icon
import androidx.compose.material3.Switch
import androidx.compose.material3.SwitchDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(50.dp),
) {
SwitchExample()
}
}
}
}
}
@Composable
fun SwitchExample() {
var checked by remember { mutableStateOf(false) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = if (checked) {
// thumb content when switch is checked
null
} else {
{
Icon(
imageVector = Icons.Filled.Lock,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
}
)
}
Screenshot
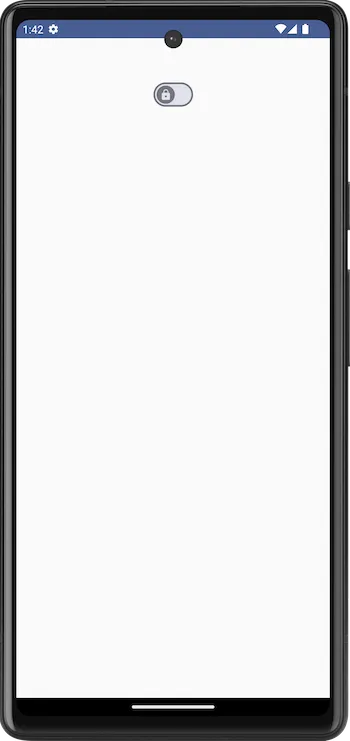
Summary
In this tutorial, we have seen how to set the thumb content for a Switch
composable when the switch is in unchecked state, in Android Jetpack compose, using an example application.