Switch thumb content in Android Jetpack Compose
We set a specific content in thumb of a Switch
composable.
To set specific thumb content to the switch in Android Jetpack Compose, you can assign a composable to thumbContent
parameter of the Switch
composable.
For example, let us set an Icon (“Icons.Default.Favorite”) as content to the thumb content of the switch.
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = {
Icon(
imageVector = Icons.Default.Favorite,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
)
Screenshot when the switch is checked:
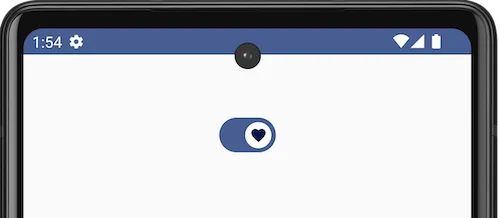
Screenshot when the switch is unchecked:
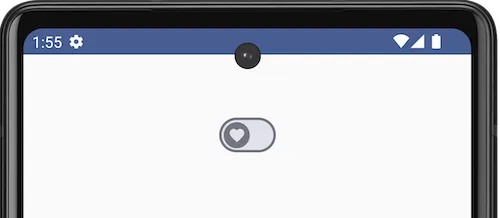
You can also set different thumb content for checked state and unchecked state using an if else statement.
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = if (checked) {
{
Icon(
imageVector = Icons.Default.Favorite,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
} else {
{
Icon(
imageVector = Icons.Default.Lock,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
}
)
Screenshot when the switch is checked:
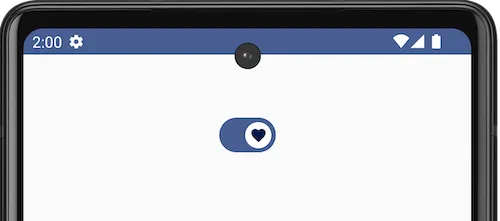
Screenshot when the switch is unchecked:
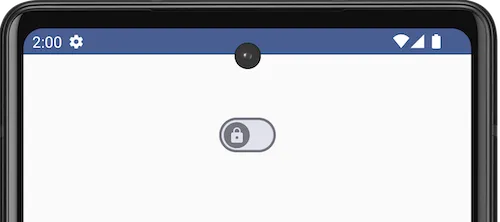
Example 1: Android Application for Switch thumb content
Now, let us create an Android application with Switch
composable, where we set the thumb content of the switch.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Favorite
import androidx.compose.material3.Icon
import androidx.compose.material3.Switch
import androidx.compose.material3.SwitchDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(50.dp),
) {
SwitchExample()
}
}
}
}
}
@Composable
fun SwitchExample() {
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = {
Icon(
imageVector = Icons.Default.Favorite,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
)
}
Screenshots
Screenshot when the switch is checked:
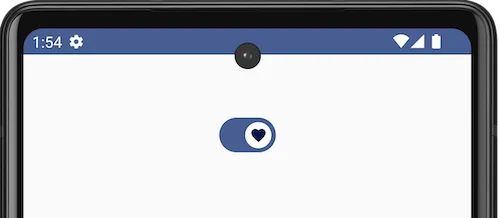
Screenshot when the switch is unchecked:
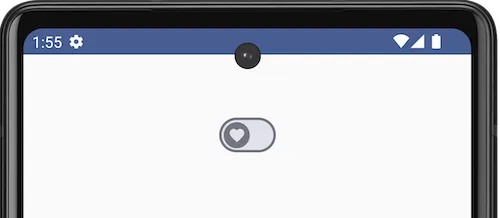
Example 2: Android Application for different Switch thumb content when checked and unchecked
Now, let us create an Android application with Switch
composable, where we set a thumb content with a Favorite Icon when the switch is checked, and we set a different thumb content like a Lock Icon when the switch is unchecked.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Favorite
import androidx.compose.material.icons.filled.Lock
import androidx.compose.material3.Icon
import androidx.compose.material3.Switch
import androidx.compose.material3.SwitchDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(50.dp),
) {
SwitchExample()
}
}
}
}
}
@Composable
fun SwitchExample() {
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = {
checked = it
},
thumbContent = if (checked) {
{
Icon(
imageVector = Icons.Default.Favorite,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
} else {
{
Icon(
imageVector = Icons.Default.Lock,
contentDescription = null,
modifier = Modifier.size(SwitchDefaults.IconSize),
)
}
}
)
}
Screenshots
Screenshot when the switch is checked:
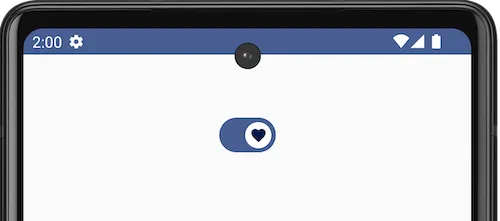
Screenshot when the switch is unchecked:
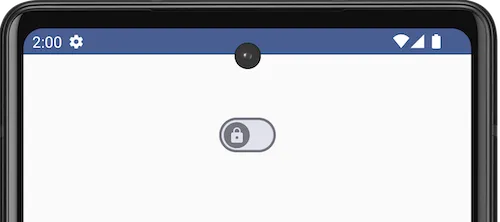
Summary
In this tutorial, we have seen how to set the thumb content for a Switch
composable, with the help of an example application. Also, we have seen how to set different thumb content for the checked and unchecked states.