Top Bar in Scaffold
The Top Bar in a Scaffold usually contains the title of the screen and navigation controls. It’s commonly used for branding, screen titles, navigation, and actions.
Syntax of Top Bar in the Scaffold
Here is a basic syntax example of a top bar within a Scaffold:
Scaffold(
topBar = {
TopAppBar(
title = { Text("Top Bar Title") },
actions = {
// Define actions here
}
)
},
content = { /* Content goes here */ }
)
Example Application
In this example, we shall go through a basic application which uses a Scaffold, where the Scaffold contains title and some actions.
Create an Android application with Compose, and use the following code in your MainActivity.kt file.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Favorite
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Icon
import androidx.compose.material3.IconButton
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
ScaffoldExample()
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ScaffoldExample() {
Scaffold(
topBar = {
TopAppBar(
colors = TopAppBarDefaults.centerAlignedTopAppBarColors(
containerColor = MaterialTheme.colorScheme.primaryContainer,
titleContentColor = MaterialTheme.colorScheme.primary,
),
title = {
Text("Sample Application")
},
actions = {
IconButton(onClick = { /* doSomething */ }) {
Icon(Icons.Filled.Favorite, contentDescription = "Favorite")
}
}
)
},
) { innerPadding ->
Column(
modifier = Modifier
.padding(innerPadding),
verticalArrangement = Arrangement.spacedBy(16.dp),
) {
Text(
modifier = Modifier.padding(8.dp),
text = "Hello World",
)
}
}
}
Screenshot
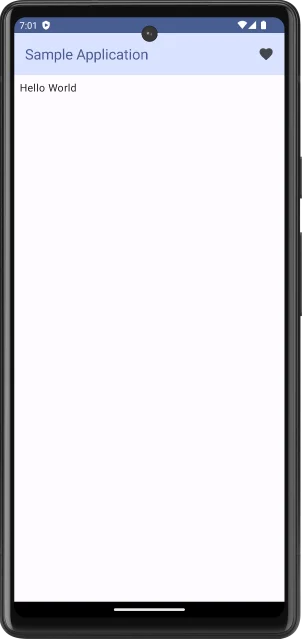