Scaffold in Android Jetpack Compose
Scaffold gives you the basic application layout based on Material Design guidelines.
Scaffold has top application bar, bottom application bar, floating action button, and many other options.
The syntax of Scaffold
composable is:
public fun Scaffold(
modifier: Modifier,
topBar: @Composable () -> Unit,
bottomBar: @Composable () -> Unit,
snackbarHost: @Composable () -> Unit,
floatingActionButton: @Composable () -> Unit,
floatingActionButtonPosition: FabPosition,
containerColor: Color,
contentColor: Color,
contentWindowInsets: WindowInsets,
content: @Composable (PaddingValues) -> Unit
): Unit
In this tutorial, we shall go through a basic example of a Scaffold, where we define a Scaffold with some basic top bar, bottom bar, floating action button, and some content.
Create an Android application with Compose, and use the following code in your MainActivity.kt file.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material3.BottomAppBar
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.FloatingActionButton
import androidx.compose.material3.Icon
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.runtime.getValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
ScaffoldExample()
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ScaffoldExample() {
var presses by remember { mutableStateOf(0) }
Scaffold(
topBar = {
TopAppBar(
colors = TopAppBarDefaults.centerAlignedTopAppBarColors(
containerColor = MaterialTheme.colorScheme.primaryContainer,
titleContentColor = MaterialTheme.colorScheme.primary,
),
title = {
Text("Top app bar")
}
)
},
bottomBar = {
BottomAppBar {
Text(
modifier = Modifier
.fillMaxWidth(),
textAlign = TextAlign.Center,
text = "Bottom app bar",
)
}
},
floatingActionButton = {
FloatingActionButton(onClick = { presses++ }) {
Icon(Icons.Default.Add, contentDescription = "Add")
}
}
) { innerPadding ->
Column(
modifier = Modifier
.padding(innerPadding),
verticalArrangement = Arrangement.spacedBy(16.dp),
) {
Text(
modifier = Modifier.padding(8.dp),
text = "Scaffold content"
)
}
}
}
At the time of writing this tutorial, Scaffold is still experimental. Therefore we have used @OptIn(ExperimentalMaterial3Api::class)
before ScaffoldExample
composable in the above code.
The topBar
is used to display the title of the application.
The bottomBar
can be used to display navigation, or some actions. But in this example, we shall display some dummy text, just to keep the example simple for Scaffold.
Then we have a Floating Action Button (FAB), that floats on the bottom right corner just above the bottom bar. Also, we have added some functionality to this button, such that when user presses this FAB, we use a counter to increment the number of presses and display it in the Scaffold body.
Run the app, and the Scaffold looks something like the following screenshot.
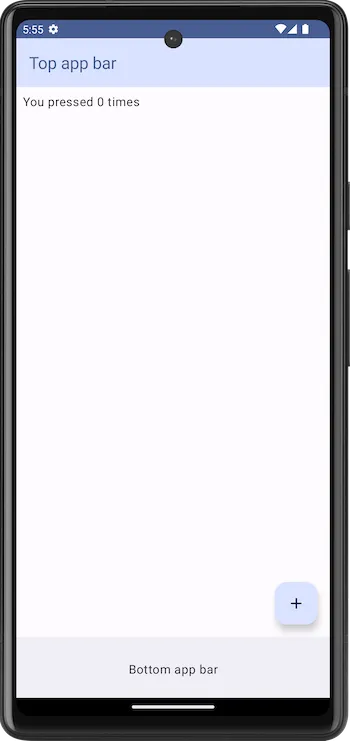
When user presses the FAB, the counter is incremented and the count is shown. This is kind of a basic demonstration to the state management of the application, where we can access some values during the app usage.
Consider that user pressed the FAB five times.
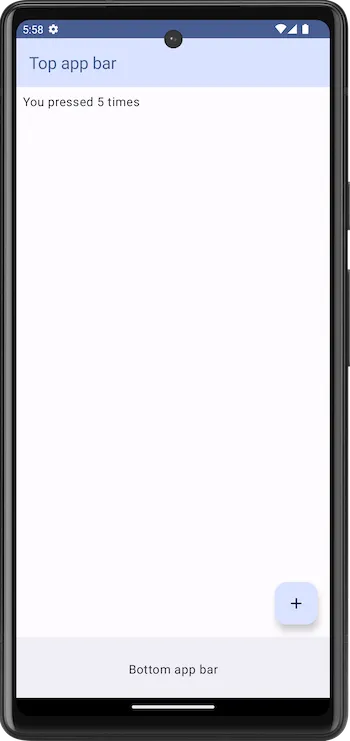
Summary
Concluding this tutorial, we have seen what a Scaffold is, how to use Scaffold in an Android application, with an example.