Android Jetpack Compose – Image with Rounded Corners
We can display an image with rounded corners, in Android Jetpack Compose.
To display an image with rounded corners using Image composable in Android Jetpack Compose, you can use Modifier.clip() with the modifier parameter of the image composable. Clip the image with RoundedCornerShape with a specific roundness in terms of percentage of length value.
The following is a simple code snippet to display an image with rounded corners of 10% the size of image, using Image composable.
Image(
modifier = Modifier
.clip(RoundedCornerShape(10))
)
You can also specify the size of round in pixels.
Image(
modifier = Modifier
.clip(RoundedCornerShape(10.sp))
)
Example Android Application for Image with Rounded Corners
In the following example, we display the following image, with rounded corners using RoundedCornerShape() with a round of 10% the size of image.
sample-image.jpg
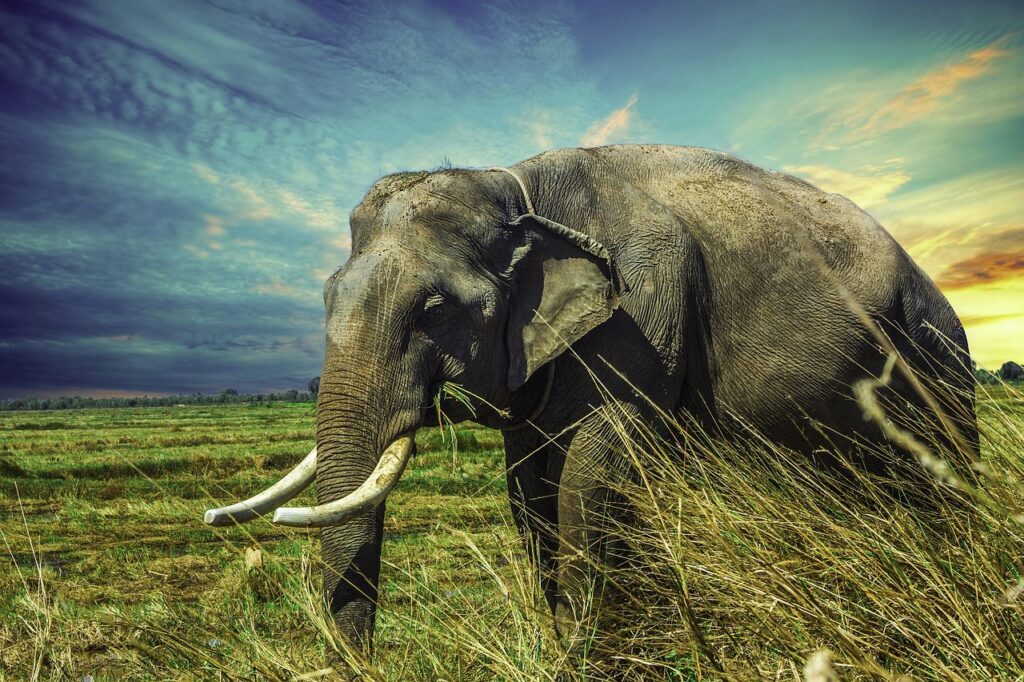
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(20.dp),
) {
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
modifier = Modifier
.clip(RoundedCornerShape(10))
)
}
}
}
}
}
Screenshot

Let us change the size of round for the image corners to 50.dp, i.e., 50 display pixels.
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
modifier = Modifier
.clip(RoundedCornerShape(50.dp))
)
Screenshot

You can play with the size of roundedness, as per your requirement.
Summary
In this tutorial, we have seen how to display an image with rounded corners using Image composable in Android Jetpack Compose, with an example.