Android Jetpack Compose – Image with Tint
To display an image with tint using Image composable in Android Jetpack Compose, you can use colorFilter parameter. Set colorFilter parameter with ColorFilter.tint() and pass the required tint color, and blend mode as arguments.
The following is a simple code snippet to display an image with Red tint and blend mode of Darken, using Image composable.
Image(
colorFilter = ColorFilter.tint(Color.Red, blendMode = BlendMode.Darken),
)
Example Android Application for Image with Tint
In the following example, we display the following image, with red tint using colorFilter parameter of Image composable.
sample-image.jpg
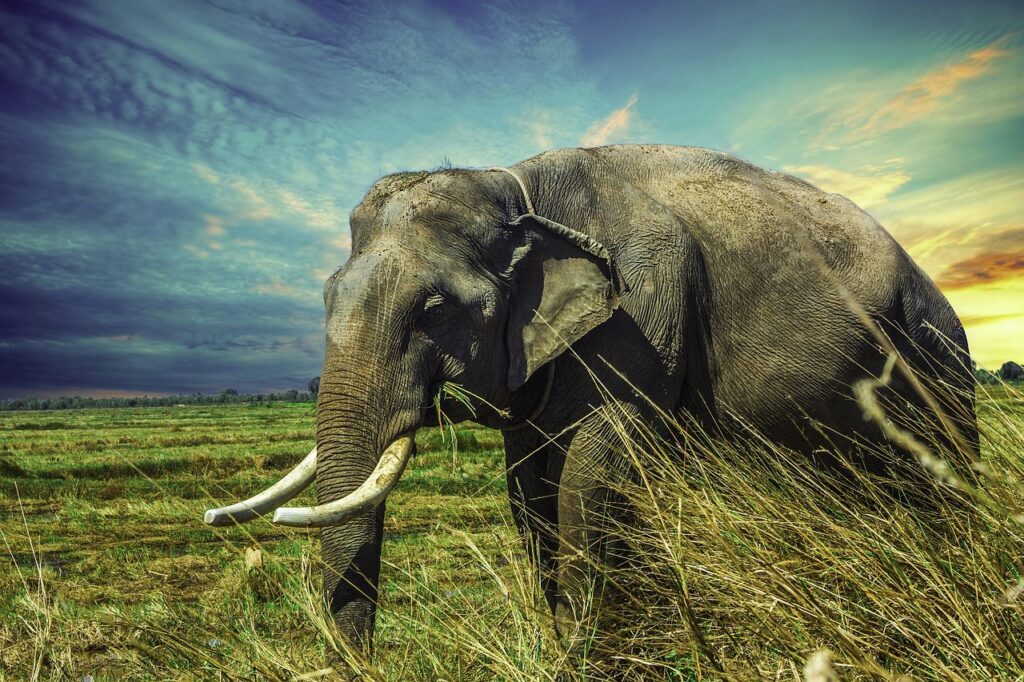
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.BlendMode
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(20.dp),
) {
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
colorFilter = ColorFilter.tint(Color.Red, blendMode = BlendMode.Darken),
)
}
}
}
}
}
Screenshot
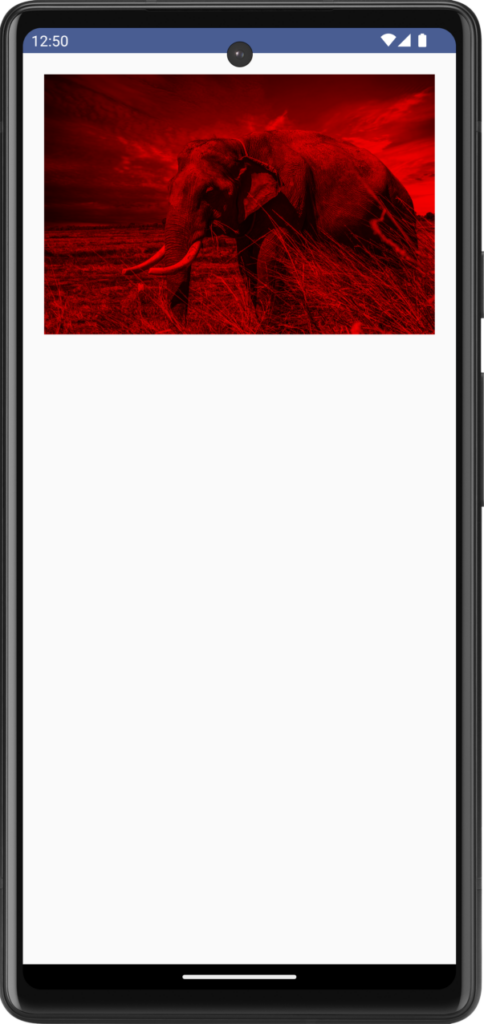
Let us change the tint color to green for the Image composable.
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
colorFilter = ColorFilter.tint(Color.Green, blendMode = BlendMode.Darken),
)
Screenshot
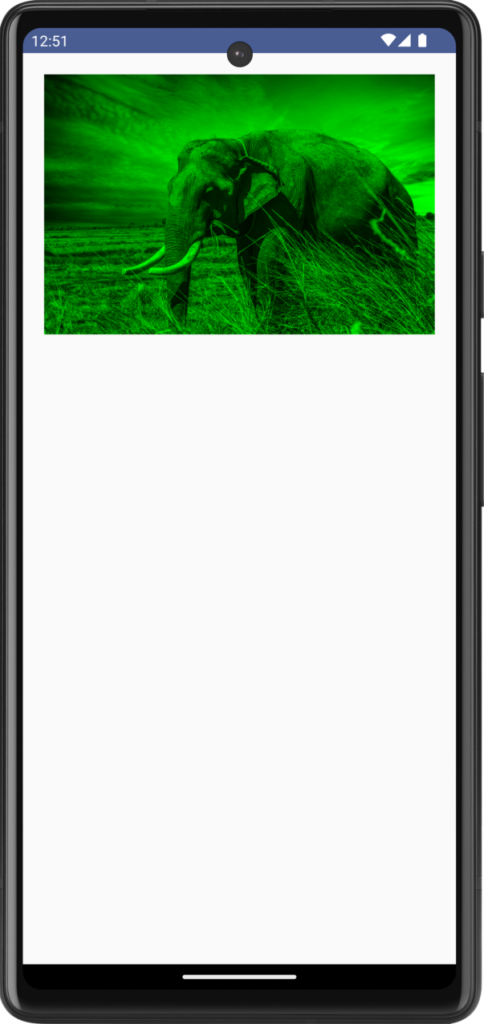
Now, let us use Green color, with a blend mode of Overlay.
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant",
colorFilter = ColorFilter.tint(Color.Green, blendMode = BlendMode.Overlay),
)
Screenshot
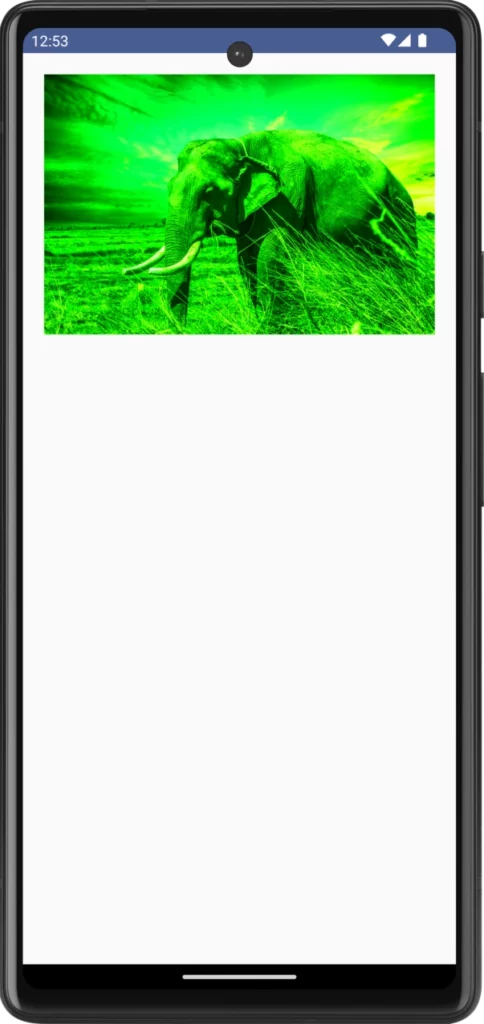
You can play with different colors, and different blend modes, and use the Color value and BlendMode as per your requirement.
Summary
In this tutorial, we have seen how to apply a tint to the image using colorFilter parameter of Image composable in Android Jetpack Compose, with an example.