FAB (Floating Action Button) Example
The Floating Action Button (FAB) is a prominent UI element in Android apps that’s typically used to display primary or high-priority action.
The FAB typically appears on the bottom right of the screen.
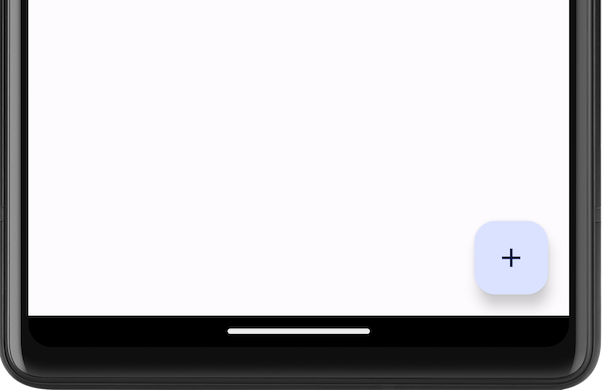
In this tutorial, we will look into an Android Jetpack Compose example, where we display FAB in the application via Scaffold, and display a Toast when user clicks on the FAB.
The following is a simple Floating Action Button that displays Add + symbol inside the FAB, and displays a Toast when user clicks on the FAB button.
FloatingActionButton(
onClick = {
Toast.makeText(
context,
"You clicked the FAB.",
Toast.LENGTH_LONG)
.show()
},
) {
Icon(Icons.Filled.Add, contentDescription = "Add")
}
Example Application for FAB (Floating Action Button)
Create an Android application with Jetpack Compose and Kotlin, and use the following MainActivity.kt code.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import android.widget.Toast
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.FloatingActionButton
import androidx.compose.material3.Icon
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.text.style.TextOverflow
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
ScaffoldExample()
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ScaffoldExample() {
Scaffold(
topBar = {
TopAppBar(
colors = TopAppBarDefaults.centerAlignedTopAppBarColors(
containerColor = MaterialTheme.colorScheme.primaryContainer,
titleContentColor = MaterialTheme.colorScheme.primary,
),
title = {
Text(
"Example Application",
maxLines = 1,
overflow = TextOverflow.Ellipsis
)
},
)
},
floatingActionButton = {
val context = LocalContext.current
FloatingActionButton(
onClick = {
Toast.makeText(
context,
"You clicked the FAB.",
Toast.LENGTH_LONG)
.show()
},
) {
Icon(Icons.Filled.Add, contentDescription = "Add")
}
},
) { innerPadding ->
Column(
modifier = Modifier
.padding(innerPadding),
verticalArrangement = Arrangement.spacedBy(16.dp),
) {
// body
}
}
}
Screenshot on Emulator
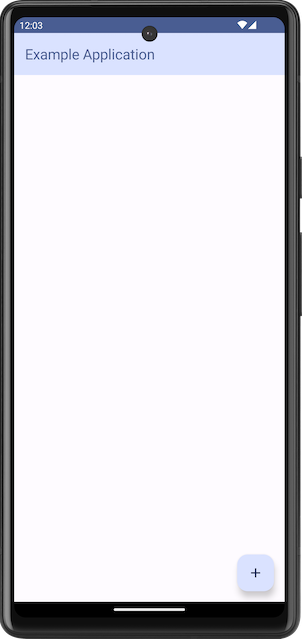
When user clicks on the FAB, a toast appears as shown in the following.
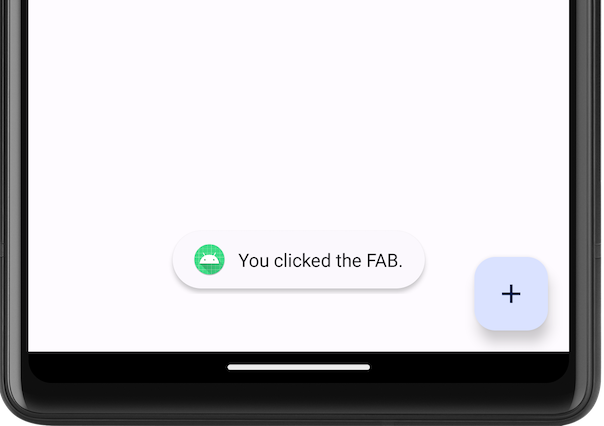
Summary
In this tutorial, we have seen how to display FAB using FloatingActionButton composable, and implement onclick code, with an example, in Android Jetpack Compose.