Android Jetpack Compose – Invert Colors of Image
We can display given image with inverted colors, in Android Jetpack Compose.
To display an image with inverted colors using Image composable in Android Jetpack Compose, take a color matrix that inverts the color of each pixel, and pass the color matrix as a filter to the colorFilter parameter of the Image composable.
The color matrix to invert each pixel of the image would be as shown in the following.
val colorMatrix = floatArrayOf(
-1f, 0f, 0f, 0f, 255f,
0f, -1f, 0f, 0f, 255f,
0f, 0f, -1f, 0f, 255f,
0f, 0f, 0f, 1f, 0f
)
And the Image composable using the above matrix to invert the image colors would be as shown in the following.
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant with Inverted Colors",
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix))
)
Example Android Application for Image with Border
In the following example, we display the following image, with inverted colors, using an Image composable.
sample-image.jpg
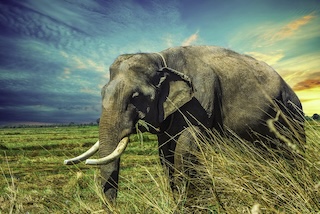
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxSize()
.padding(20.dp),
) {
val colorMatrix = floatArrayOf(
-1f, 0f, 0f, 0f, 255f,
0f, -1f, 0f, 0f, 255f,
0f, 0f, -1f, 0f, 255f,
0f, 0f, 0f, 1f, 0f
)
Image(
painter = painterResource(id = R.drawable.sample_image),
contentDescription = "Big Elephant with Inverted Colors",
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix))
)
}
}
}
}
}
Screenshot
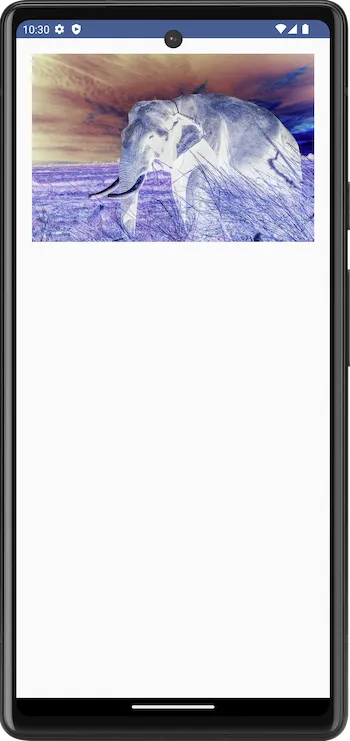
Summary
In this tutorial, we have seen how to display an image with inverted colors using Image composable in Android Jetpack Compose, with an example.